Programming
99 Artikel tersedia
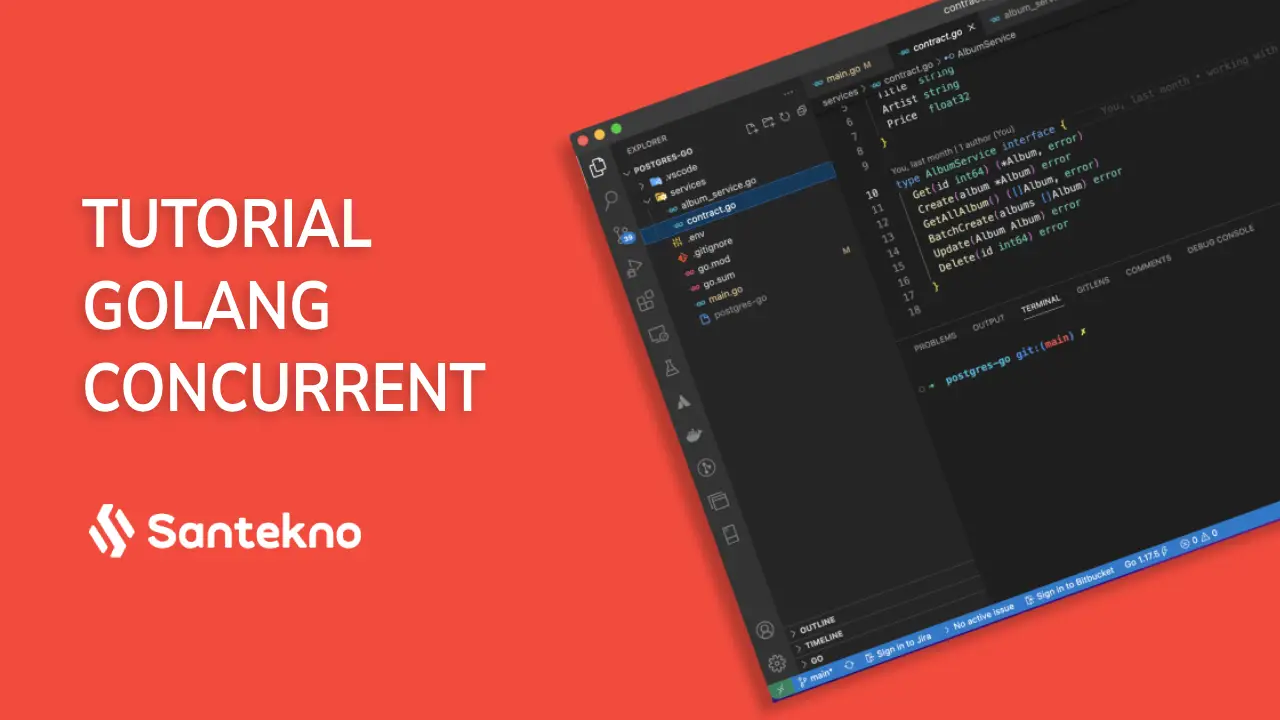
Getting to Know Sync Cond on Golang
Introduction of sync.Cond
sync.Cond
is a locking process that is used with certain conditions. sync.Cond
in the Golang synchronization package implements conditional variables that can be used in scenarios where multiple readers
are waiting for concurrent resources. Cond pooling point: multiple goroutines waiting, 1 goroutine notification event occurs. Each Cond is associated with a Lock
**(sync.Mutex or sync.RWMutex) that should be added when modifying the condition or calling the wait()
method, to protect the condition.
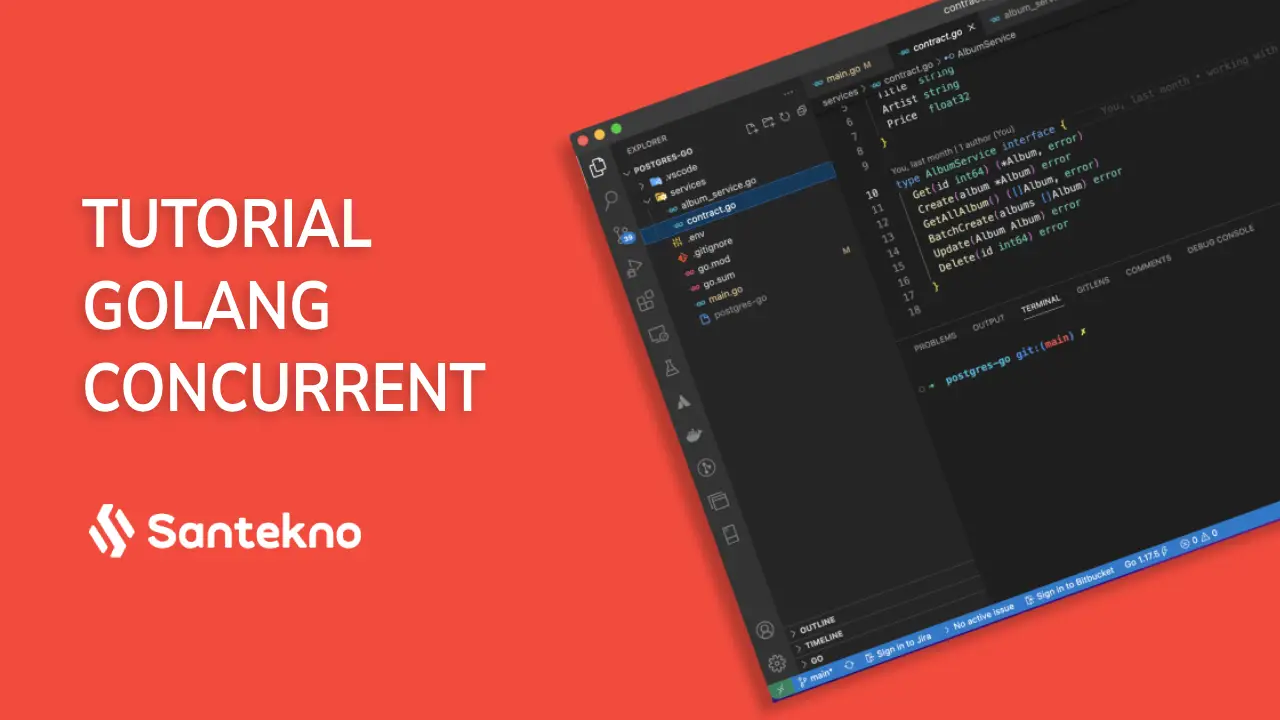
Getting to Know Sync Once On Golang
Introduction of sync.Once
We can use this feature in Golang to ensure that a function is executed only once. Sometimes if we already have many goroutines that are accessing, then with sync.Once
we can make sure that only the goroutine that has the first access can execute the function. So if there are other goroutines that are running, they will not execute and ignore the function.
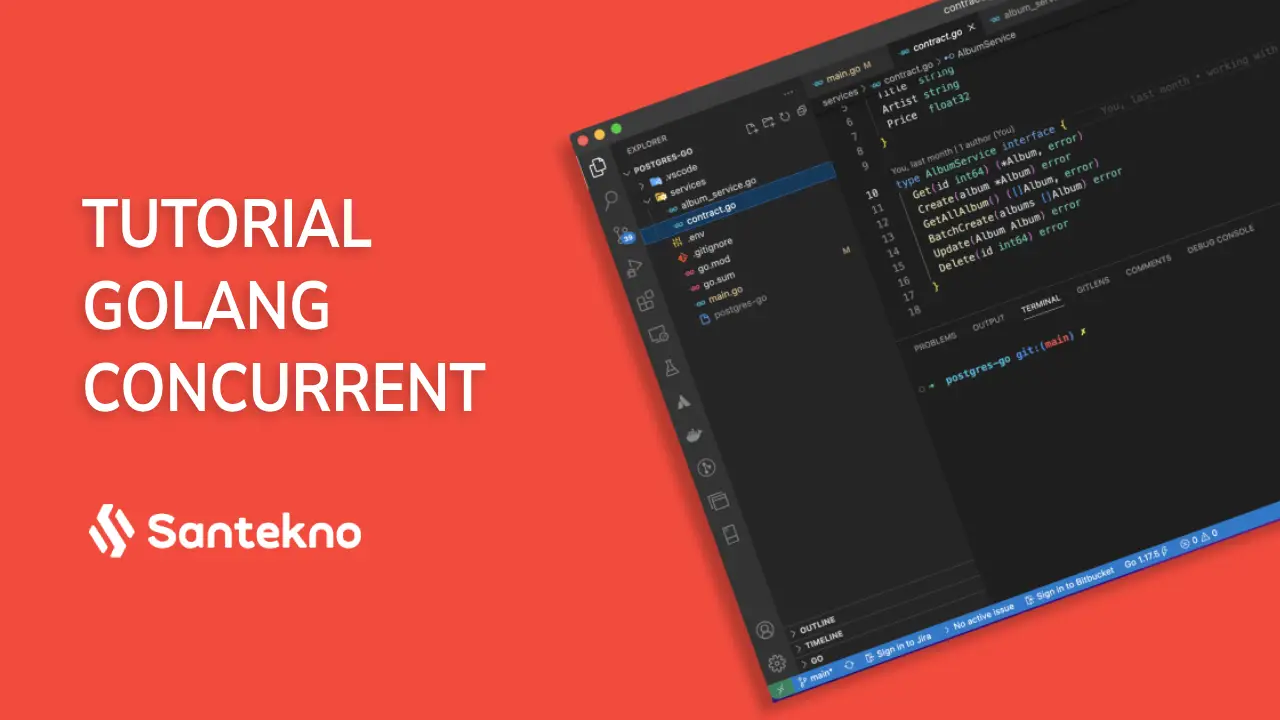
Getting to Know Sync Map on Golang
Introduction to sync.Map
This sync.Map
is actually very similar to the regular Generic Golangnya map
, but the difference is that this map
is safe to use during concurrent goroutines.
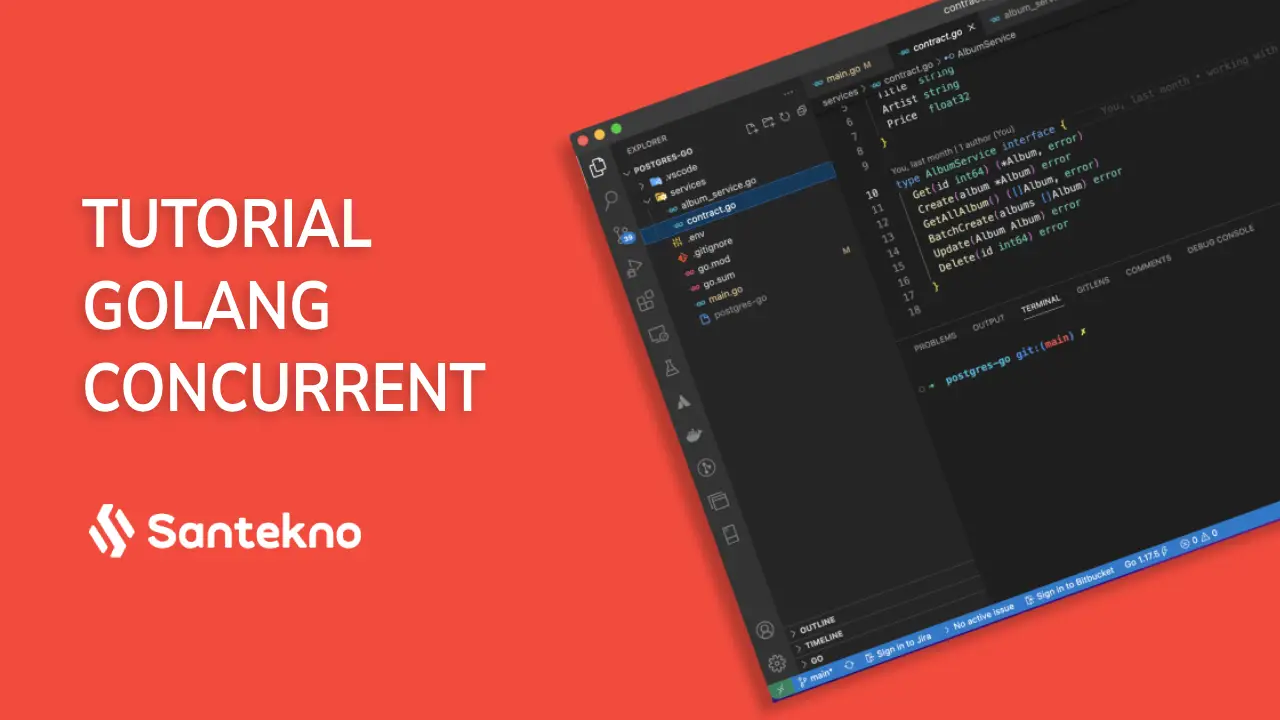
Getting to know the Sync Pool on Golang
Introduction to sync.Pool
We often hear sync.Pool when implementing a design pattern called Object Pool Pattern. A Pool is a temporary set of objects that can be stored and retrieved individually. A Pool is safe to be used by multiple goroutines simultaneously.
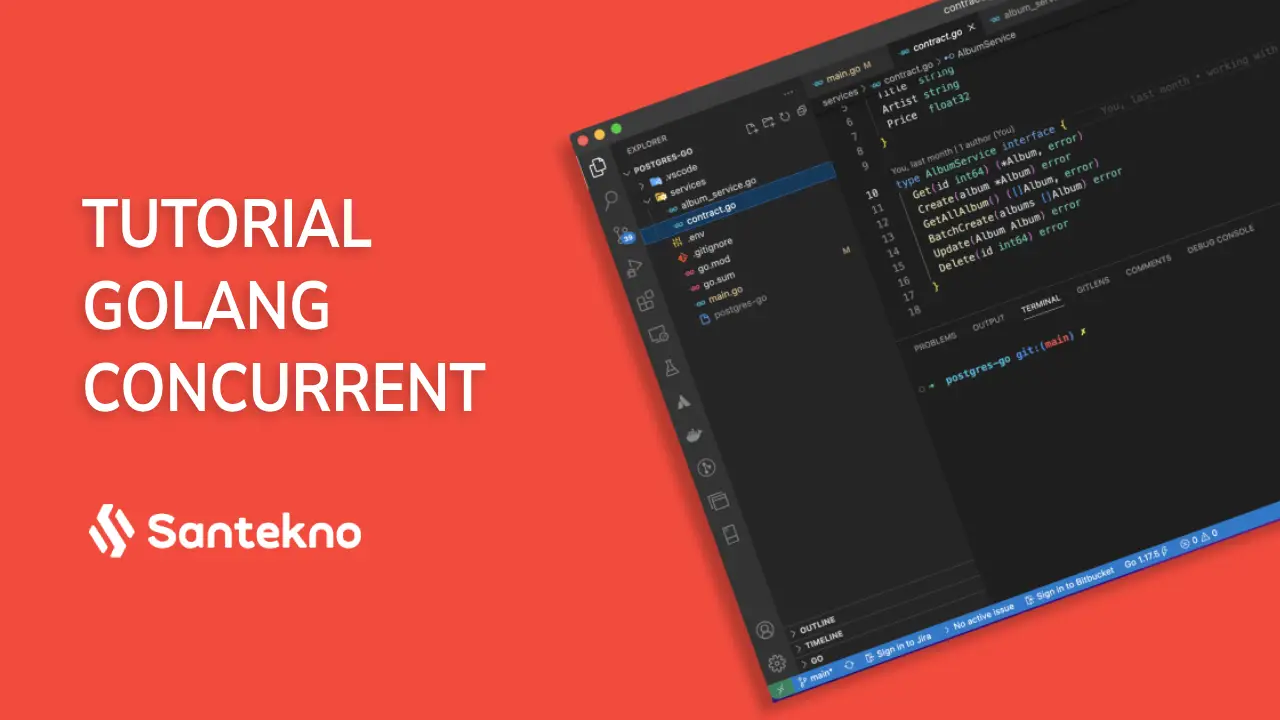
Getting to Know WaitGroup on Golang
Introduction
Waitgroup is a feature of Golang that is used to wait for a process carried out by several goroutines. This is done because we need to do the process simultaneously but when we continue the next process we need data from the previous result so we have to wait first from the previous process to get the data.
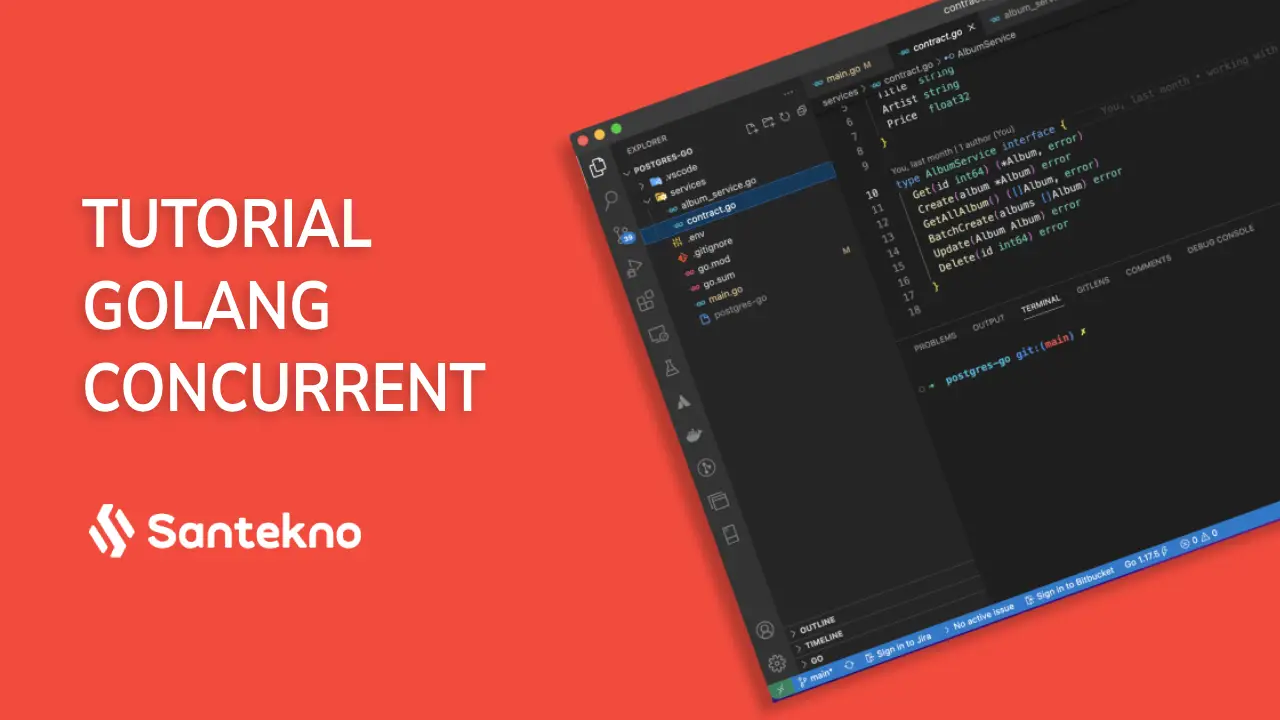
Knowing Deadlock and How to Overcome It in Golang
Introduction
One of the problems that occurs when using concurrent
or parallel
is the deadlock
system. What is deadlock? Deadlock is an event where a concurrent process or goroutine waits for each other (lock) so that none of the goroutines can run. So be careful if you create an application or program that implements mutex lock and unlock using goroutines. Well we will try directly how to simulate the golang program when there is a deadlock
.
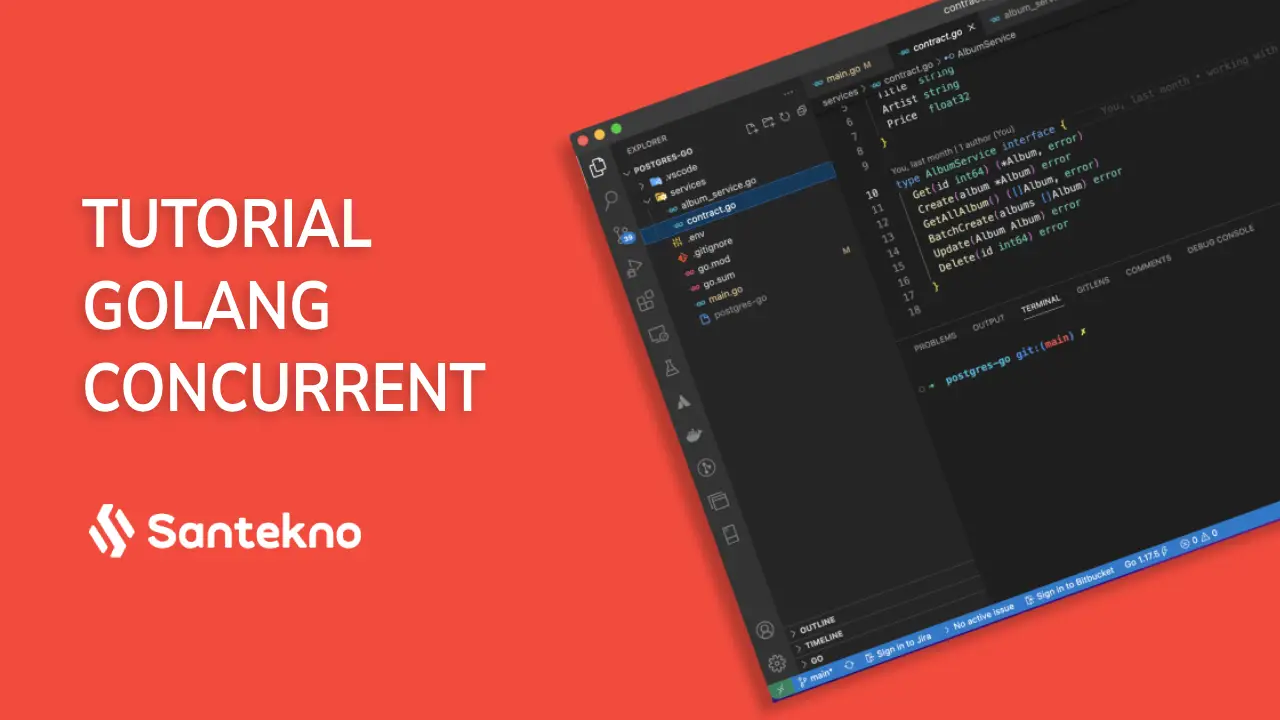
How to Create RW Mutex and Its Use in Golang
Introduction to Sync.RWMutex
After we have learned
Introduction and Creation of `Mutex` in the previous post, then we will continue to the next stage which is the introduction of RWMutex
. Now what is the difference with the previous one?
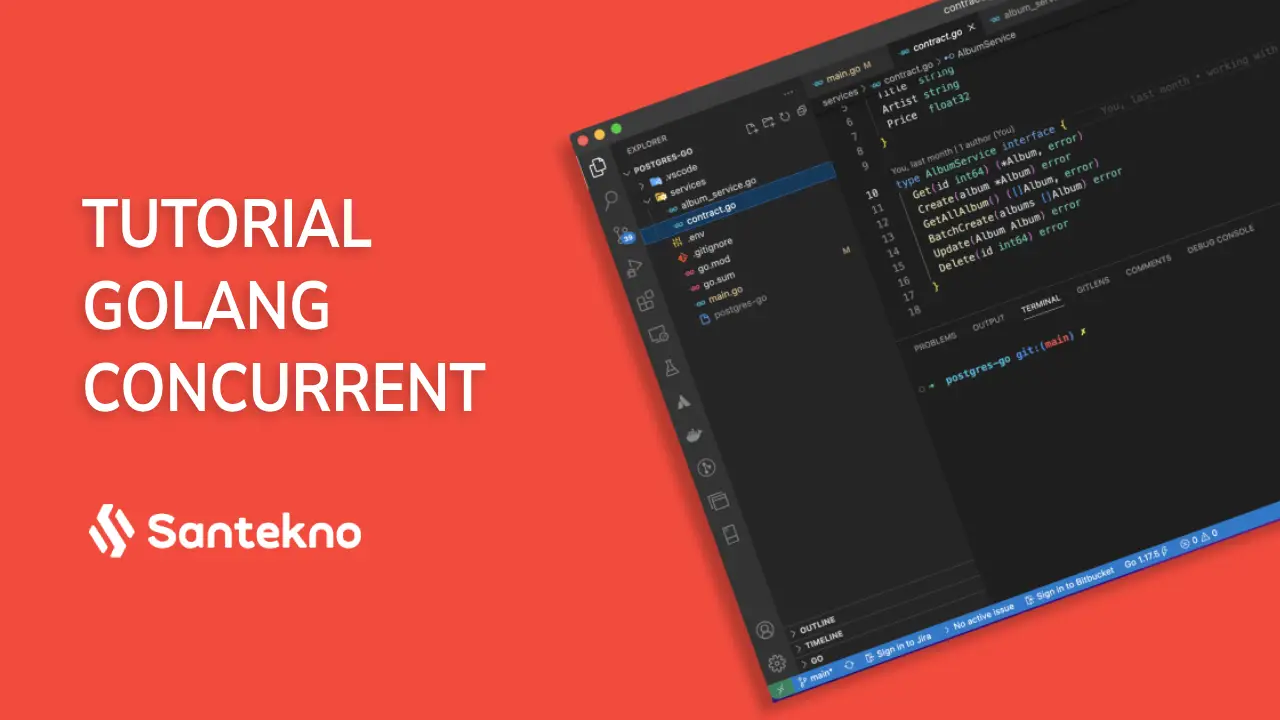
How to Create Mutex and Its Use in Golang
Introduction of Sync.Mutex
Mutex or stands for Mutual Exclusion is a way to overcome race conditions in the Golang language. Mutex can be used to do locking
and unlocking
of a mutex so that if it is locked
it will not be able to do locking
again until we do unlocking
.
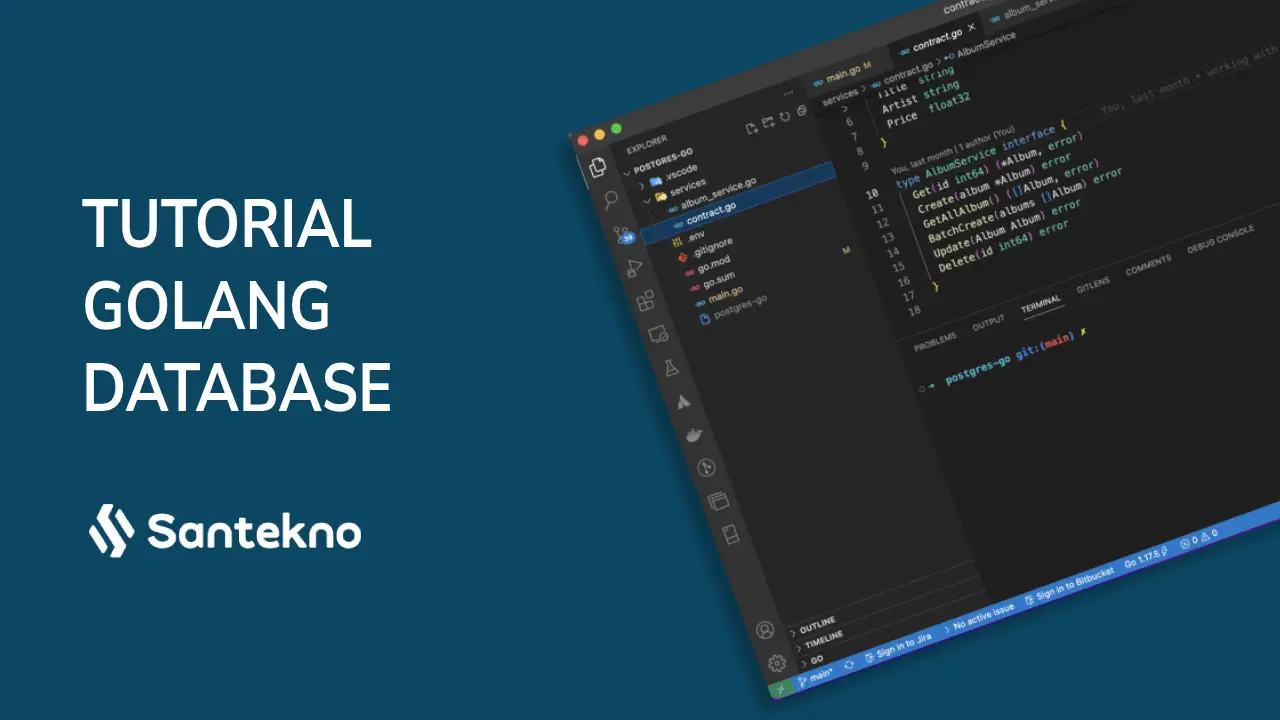
How to Communication Golang with MSSQL Server
Dependency
Make sure you have created a project with go mod init mssql-go
in the mssql-go
folder and the dependency that we will use is using
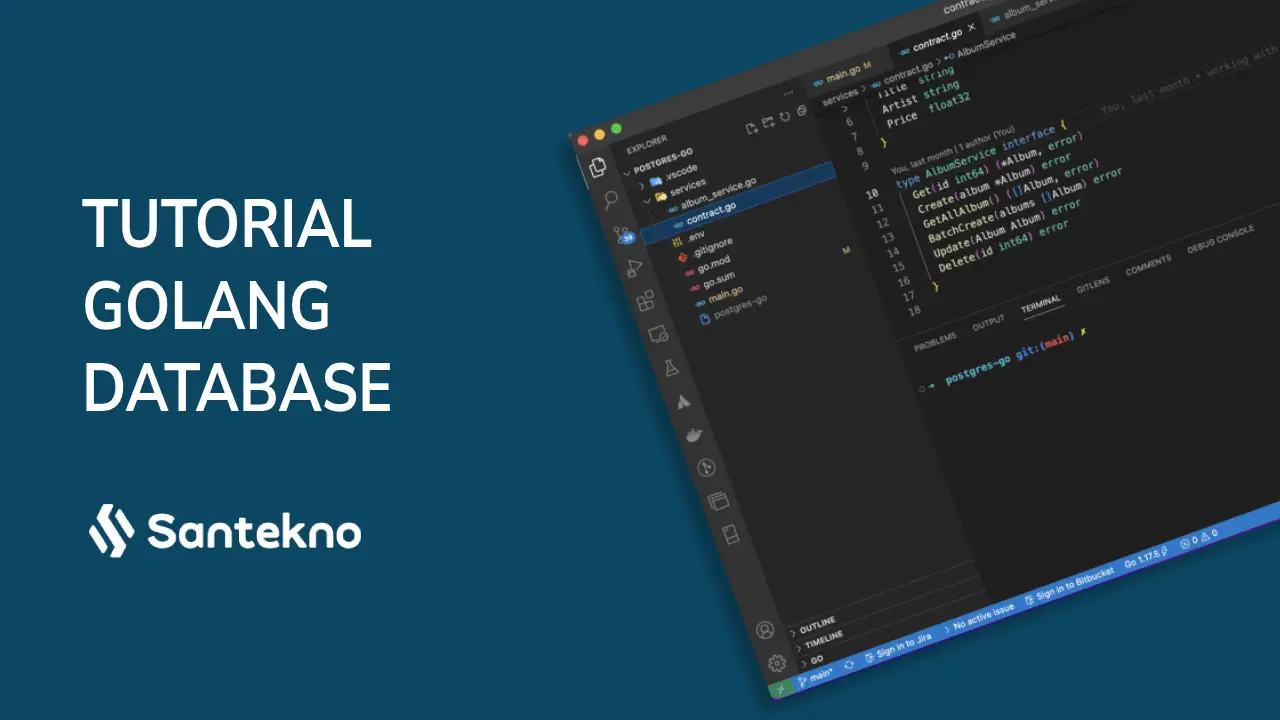
How To Communication Golang with MongoDB
Dependecy Needed
Add some dependency when we used
"go.mongodb.org/mongo-driver/bson"
"go.mongodb.org/mongo-driver/mongo"
"go.mongodb.org/mongo-driver/mongo/options"
Create a Database Connection
create database connection function into mongoDB.
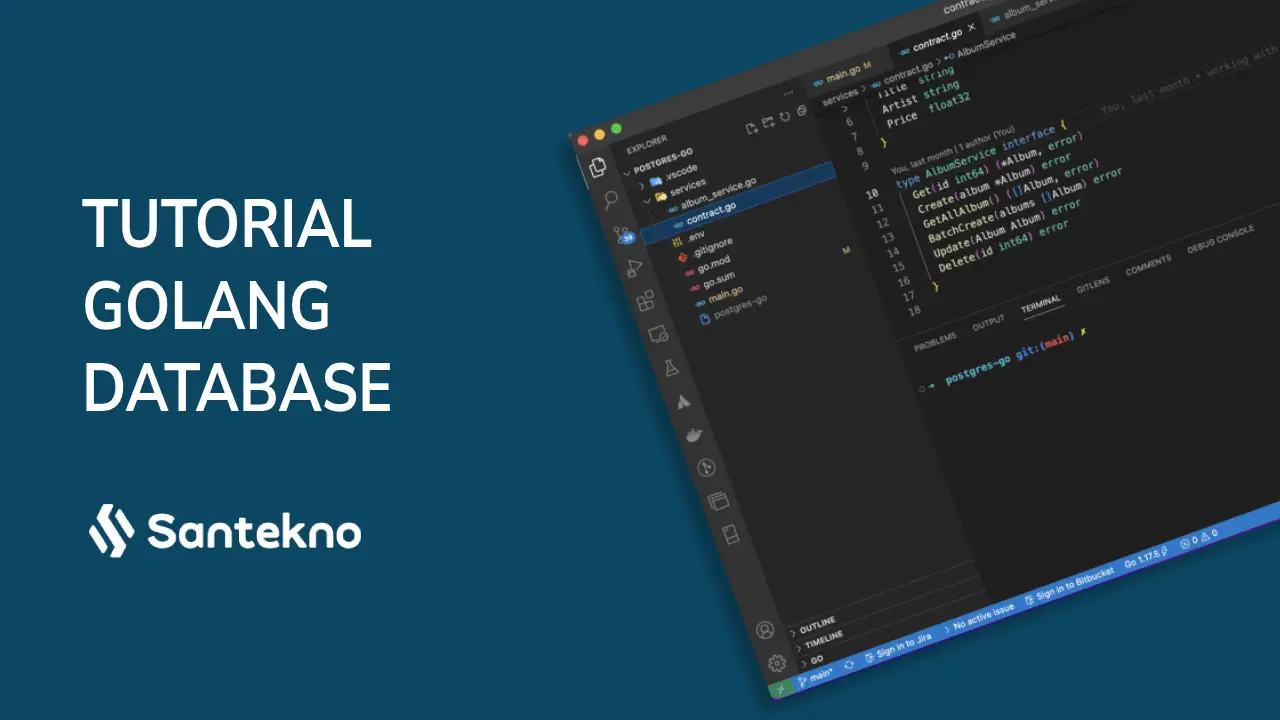
How To Communication Golang with Postgres Database
Dependency
Make sure you have created a project with go mod init postgres-go
in the postgres-go
folder and the dependency that we will use is using
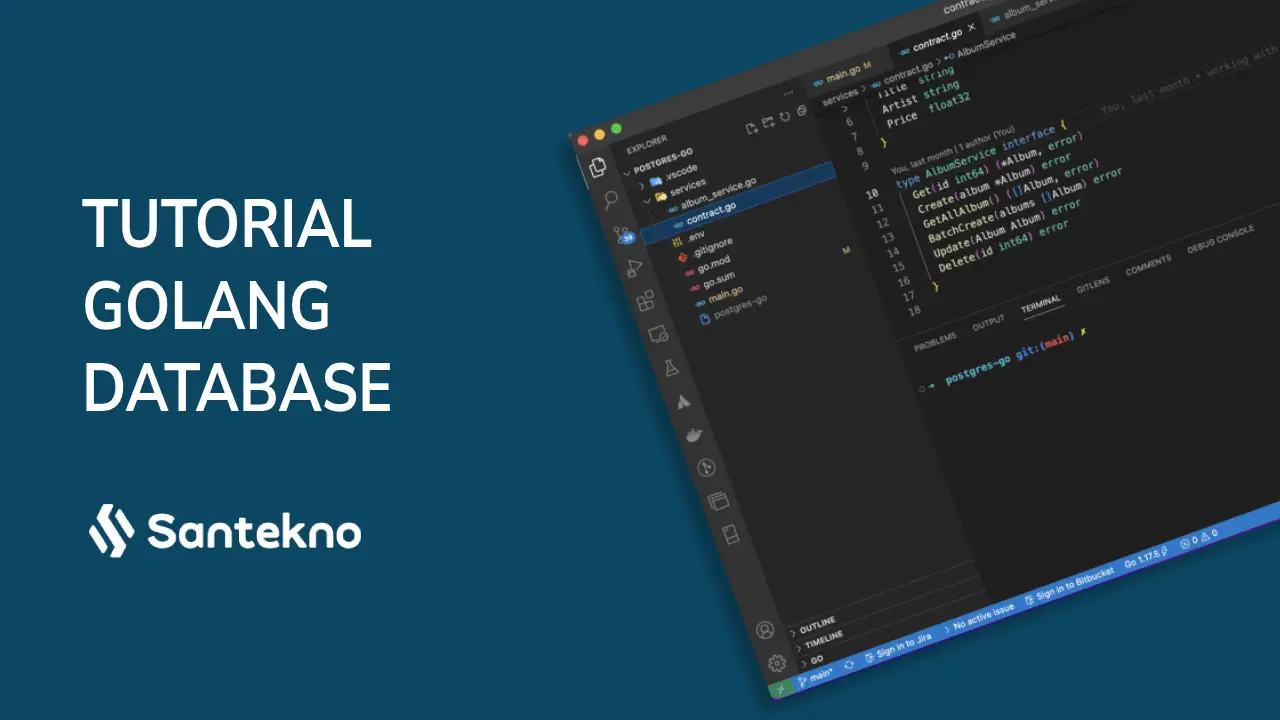
How to Communication Golang with MySQL Database
Package atau Library
import "github.com/go-sql-driver/mysql"
Project Initialization
Prepare a new folder with the name mysql-native
, then initialize the Golang module to make it more modular. Here’s a quick command.