#Concurrent
6 articles
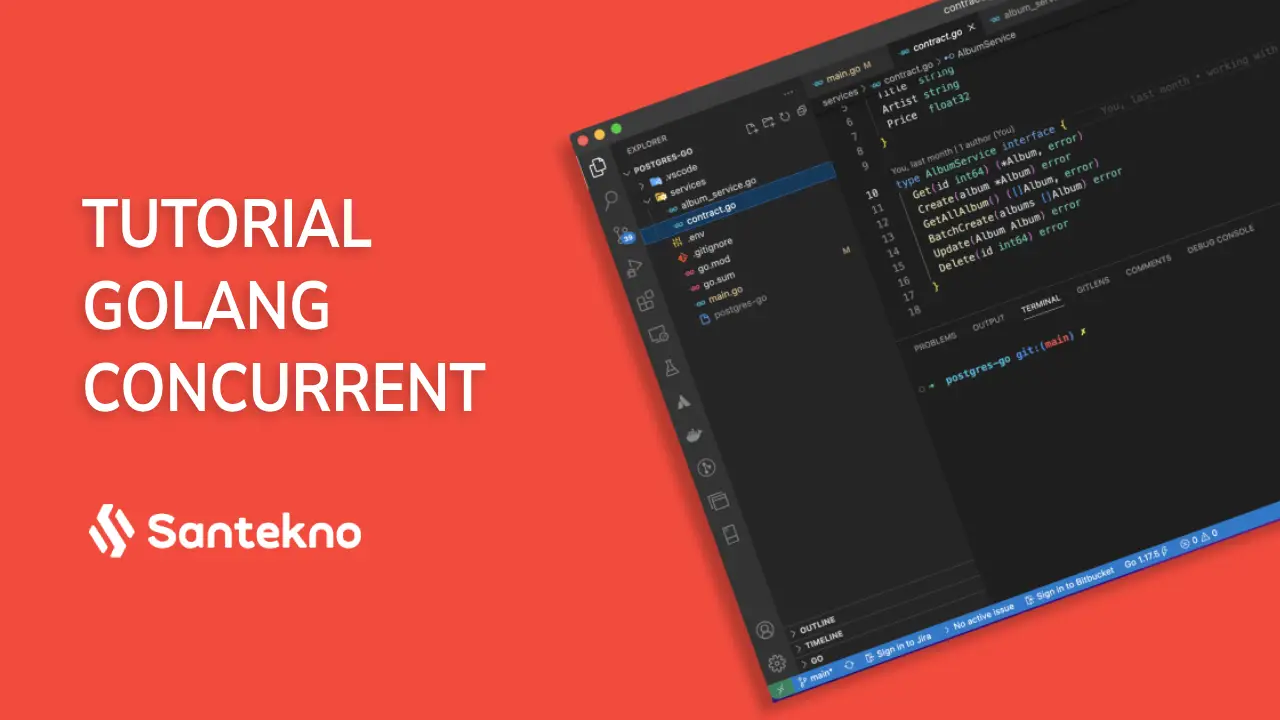
Knowing and Implementing Atomic Sync in Golang
Introduction to sync.Atomic
For atomic operations on variables in golang, the sync/atomic package offers certain low-level methods. In Go, these methods allow multiple goroutines to safely modify shared variables without using locks or other explicit synchronization. Functions like AddInt64
, AddUint32
, CompareAndSwapInt32
, etc. used to perform basic arithmetic on different types of variables can be found in the atom
package. The AddInt64
method for example, guarantees that modifications made by other goroutines will be seen when adding a certain value to an int64
variable in atomic style.
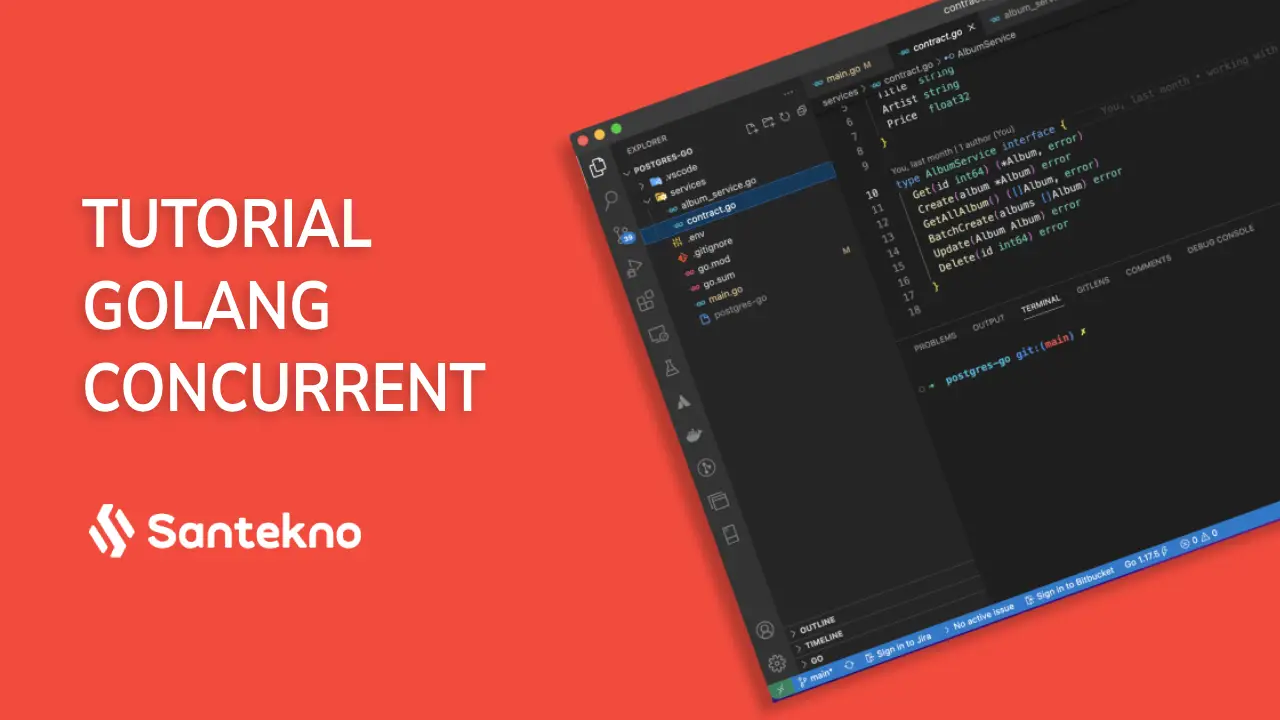
Getting to Know Sync Cond on Golang
Introduction of sync.Cond
sync.Cond
is a locking process that is used with certain conditions. sync.Cond
in the Golang synchronization package implements conditional variables that can be used in scenarios where multiple readers
are waiting for concurrent resources. Cond pooling point: multiple goroutines waiting, 1 goroutine notification event occurs. Each Cond is associated with a Lock
**(sync.Mutex or sync.RWMutex) that should be added when modifying the condition or calling the wait()
method, to protect the condition.
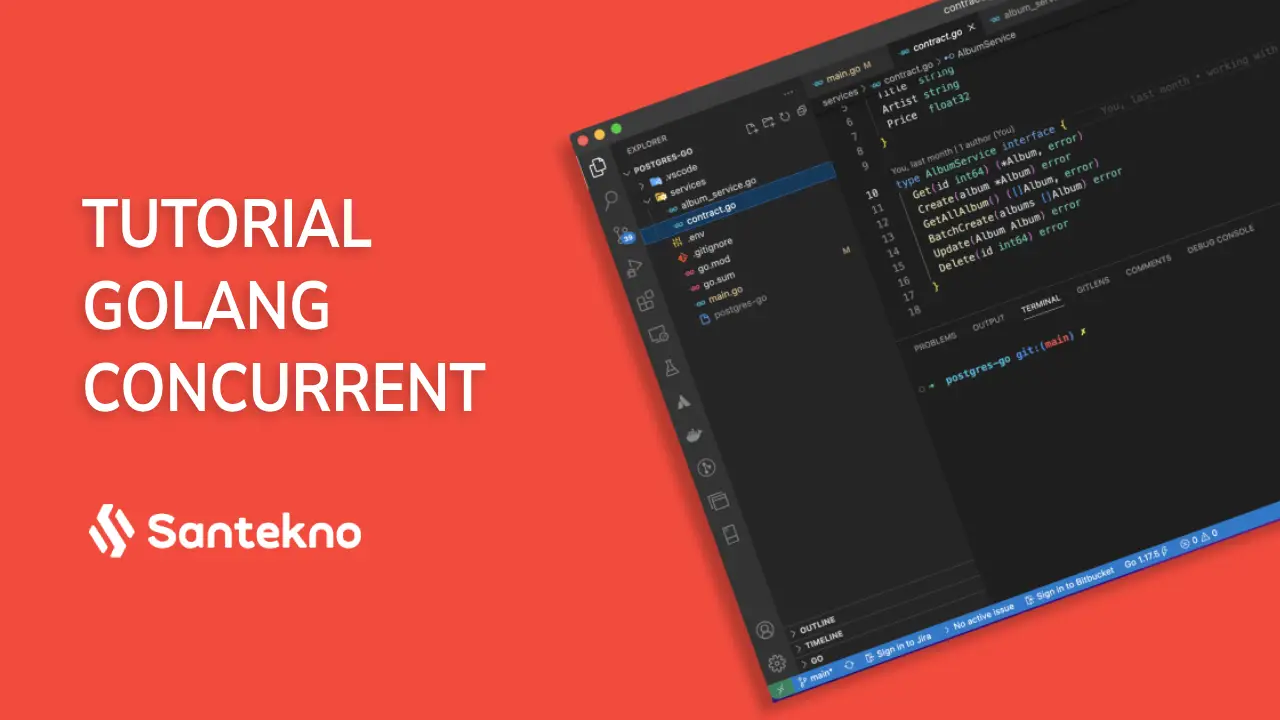
Getting to Know Sync Once On Golang
Introduction of sync.Once
We can use this feature in Golang to ensure that a function is executed only once. Sometimes if we already have many goroutines that are accessing, then with sync.Once
we can make sure that only the goroutine that has the first access can execute the function. So if there are other goroutines that are running, they will not execute and ignore the function.
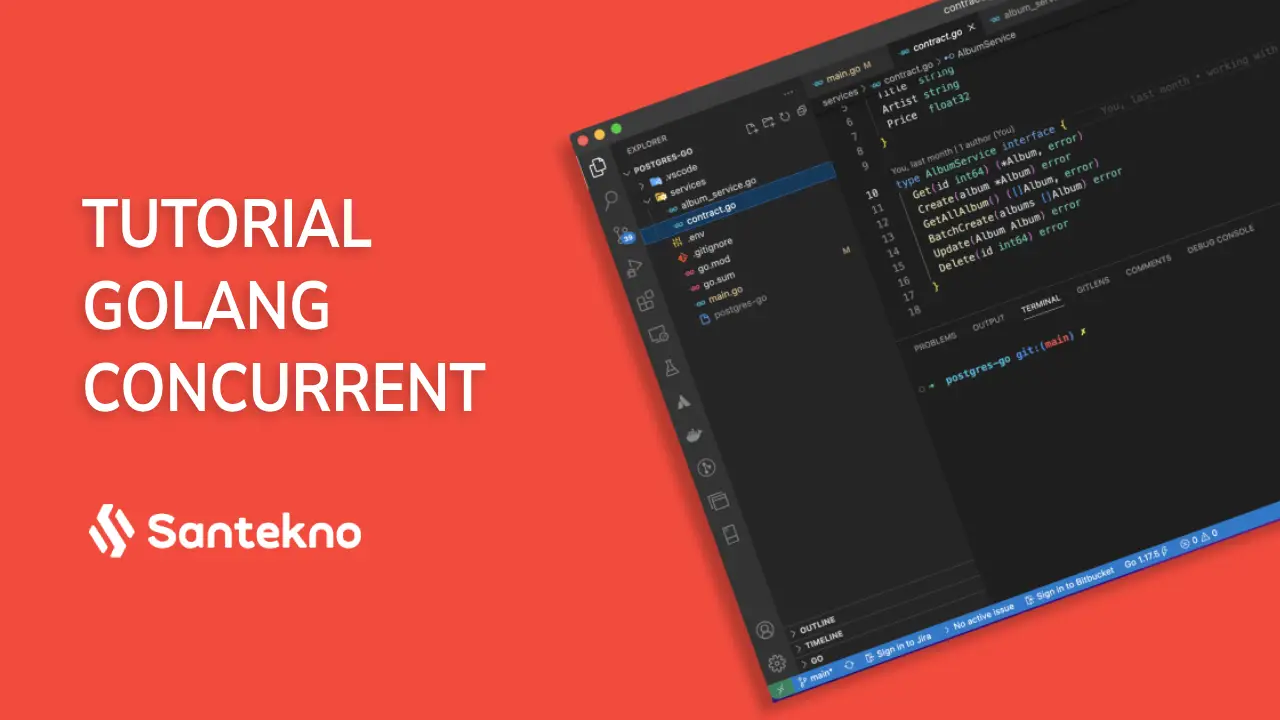
Getting to Know Sync Map on Golang
Introduction to sync.Map
This sync.Map
is actually very similar to the regular Generic Golangnya map
, but the difference is that this map
is safe to use during concurrent goroutines.
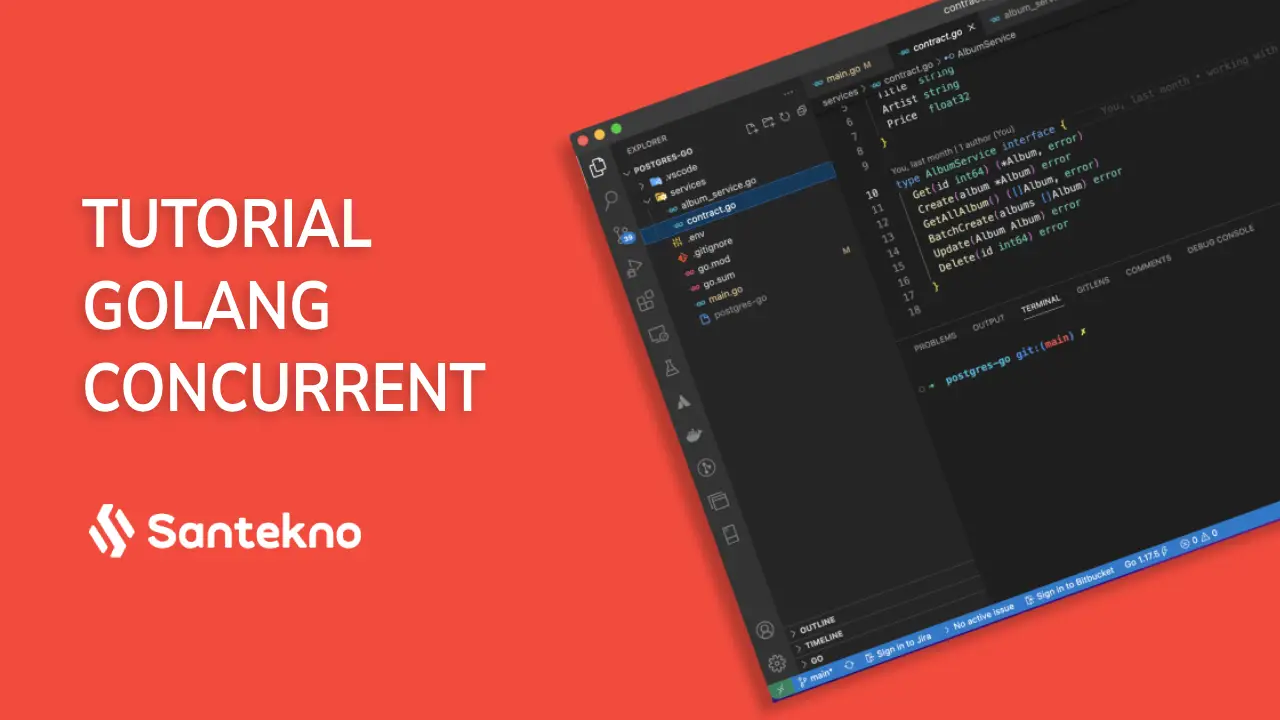
Getting to know the Sync Pool on Golang
Introduction to sync.Pool
We often hear sync.Pool when implementing a design pattern called Object Pool Pattern. A Pool is a temporary set of objects that can be stored and retrieved individually. A Pool is safe to be used by multiple goroutines simultaneously.
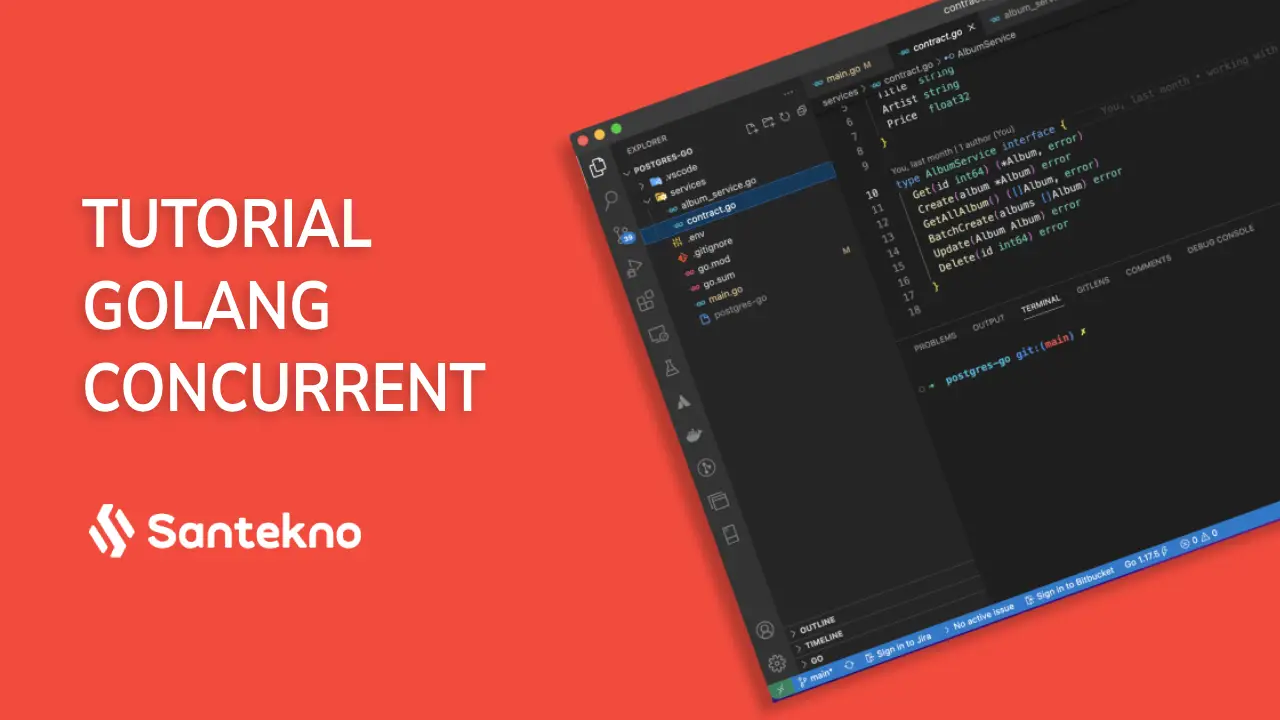
Getting to Know WaitGroup on Golang
Introduction
Waitgroup is a feature of Golang that is used to wait for a process carried out by several goroutines. This is done because we need to do the process simultaneously but when we continue the next process we need data from the previous result so we have to wait first from the previous process to get the data.