#Golang-Basic
29 articles
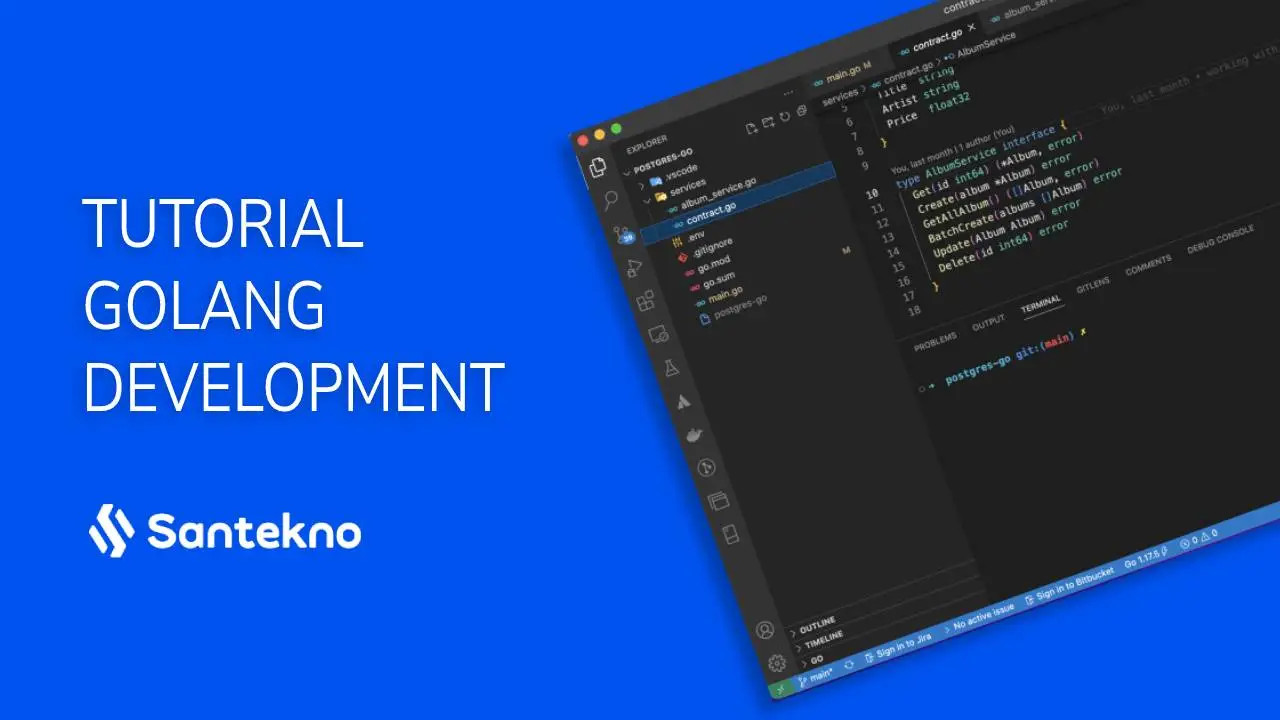
Introduction and Implementation of Golang Embed
Introduction to Embed Packages
Since Golang released version 1.16 there is a new feature called Embed. This embed package is a feature that makes it easier to open the contents of a file at compile time, automatically inserting the contents of the file into the variables that we have defined. For more details, you can see here.
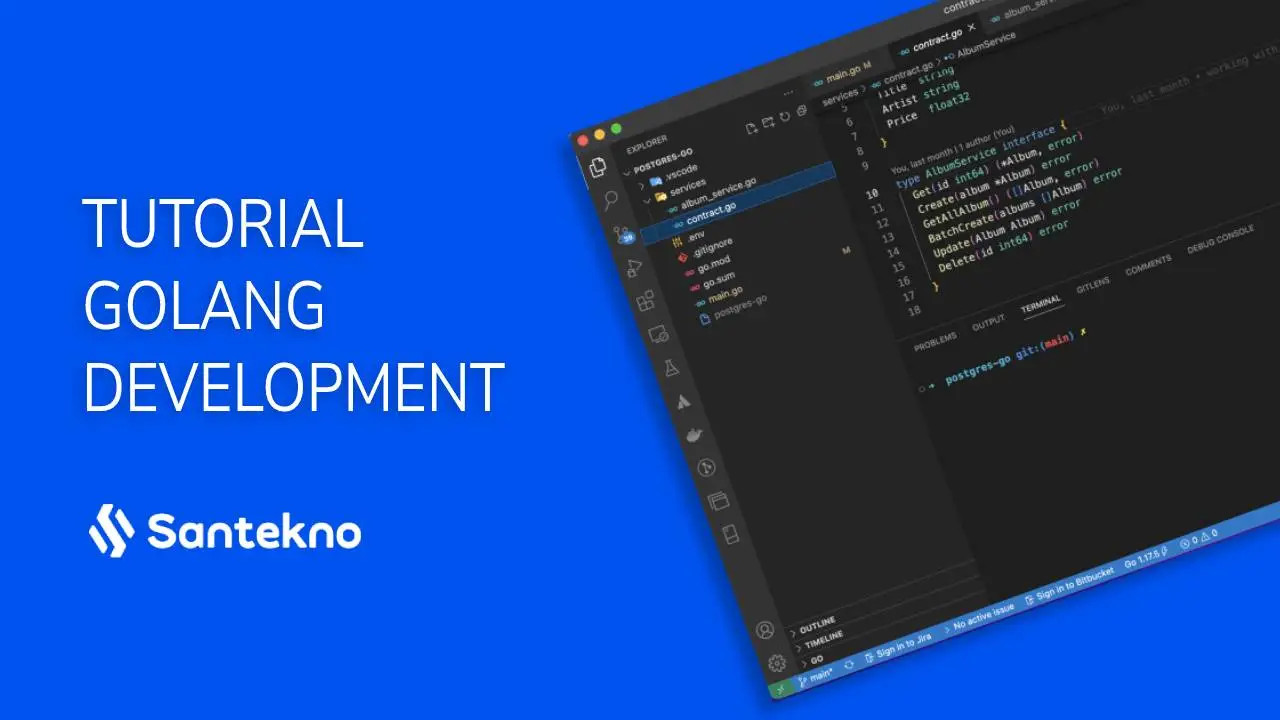
Get to know the Repository Pattern in Golang
In the book Domain-Driven Design, Eric Evans explains that
Repository is a mechanism for encapsulating storage, retrieval and search behaviour, which emulates a collection of objects
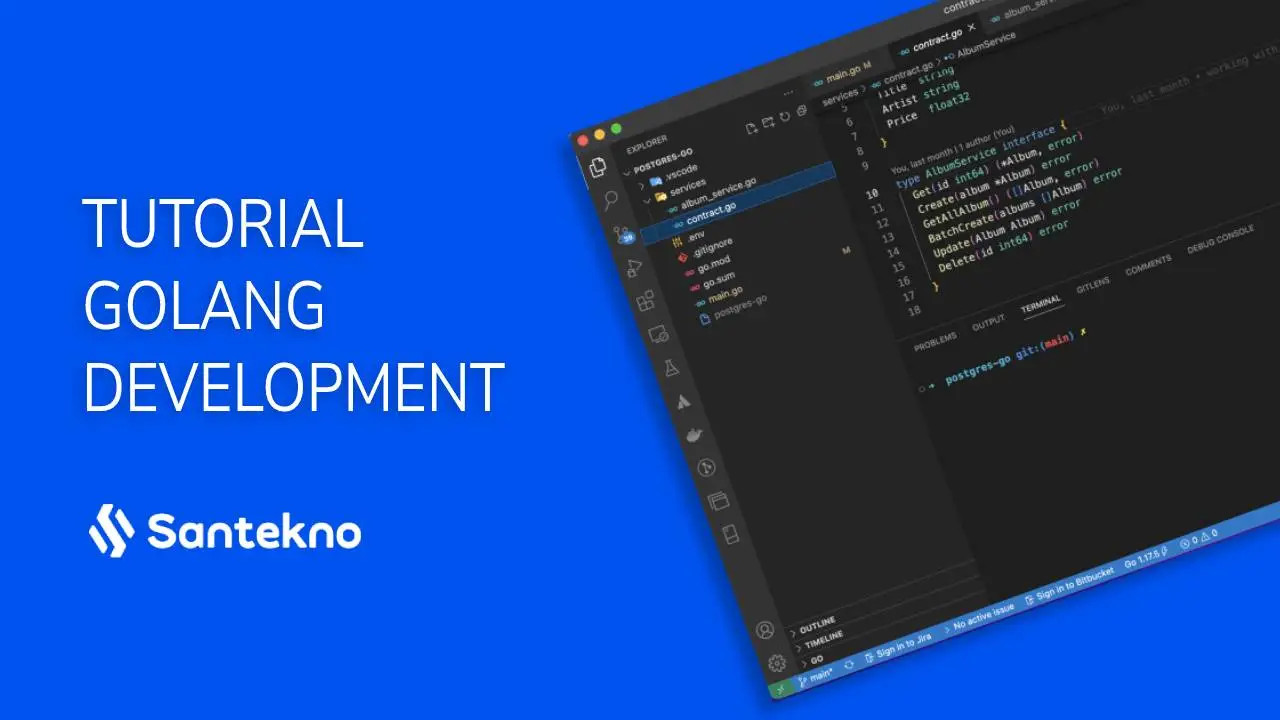
Get to know Package Context With Value in Golang
Introduction to the context.WithValue package
At the beginning of the context explanation, we know that the context will be created for the first time during initialization using context.Background()
or context.TODO()
, where the context does not have a value, aka it is still empty. We can add values from a context with a concept like a map, namely [key - value].
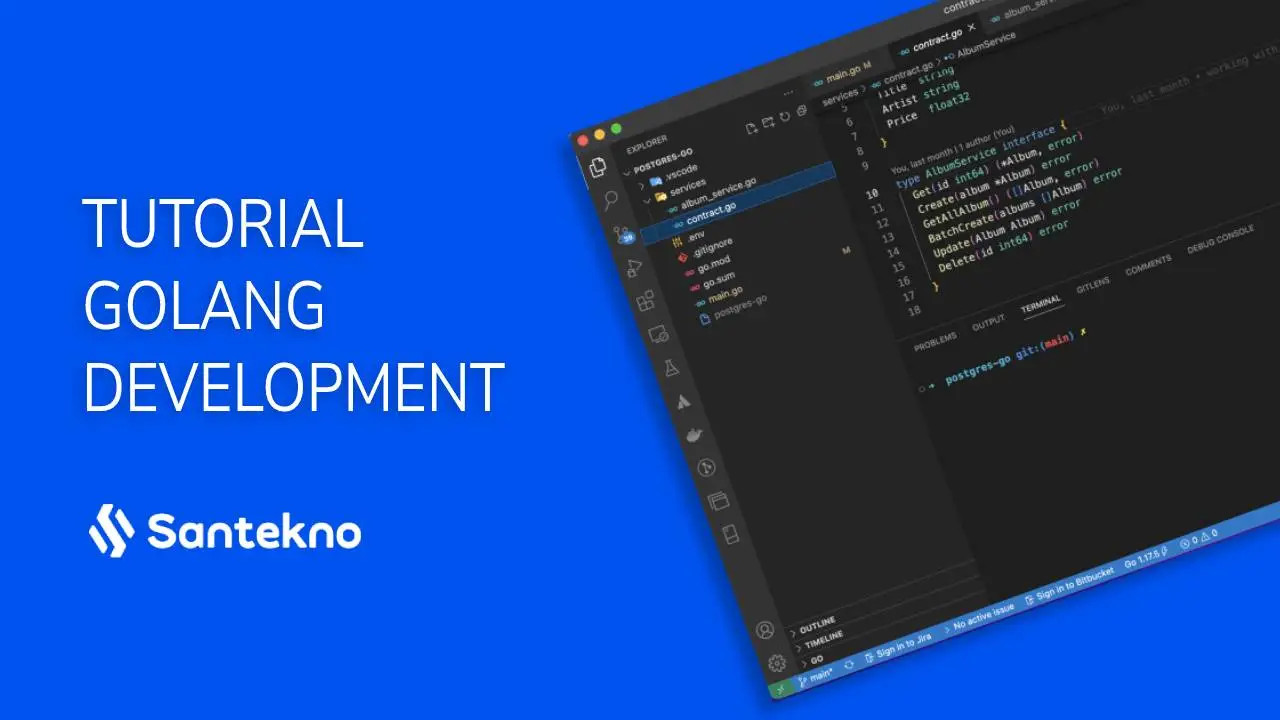
Getting to Know Package Context in Golang
Introduction to package context
Context is a package that can store and carry data values, timeout signals and deadline signals. This context runs and is created per request. The context usually carries the value from initialization until the end of the process. This context is sometimes a necessity when we code a program so that from the beginning of the function to the next function the previous value can be used if the process in the next function requires data from the initial process.
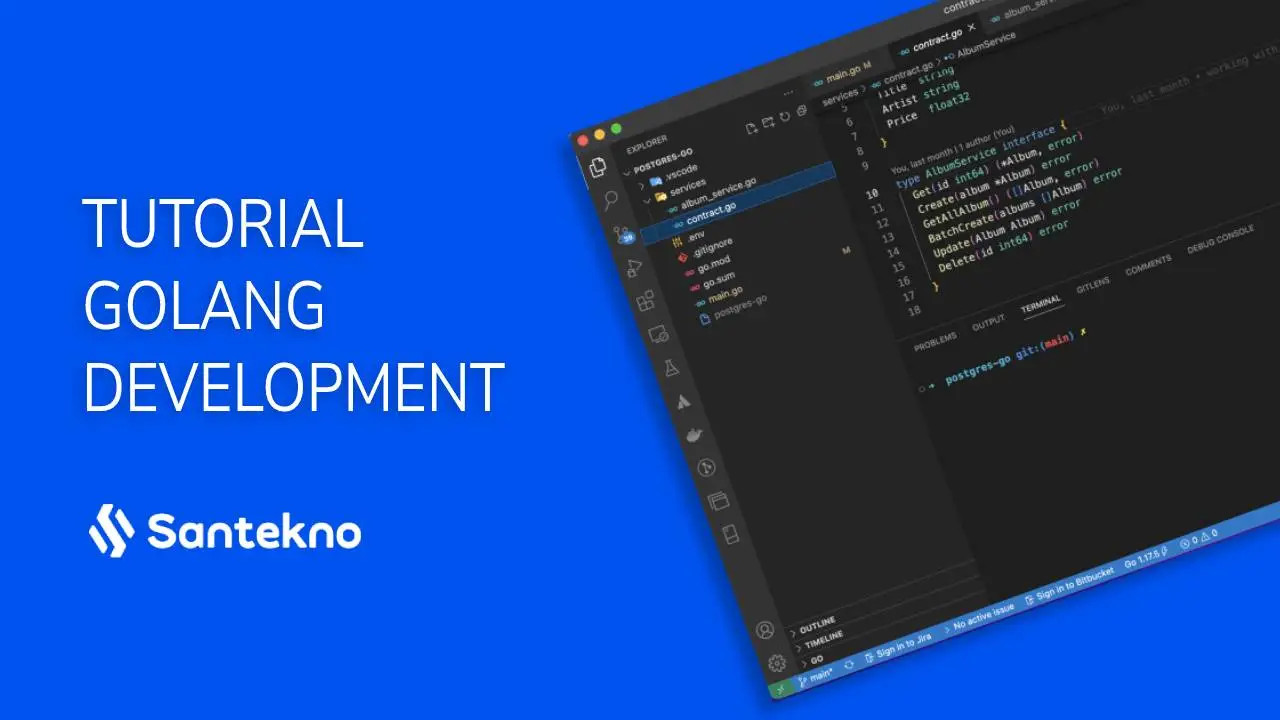
Get to know the Package Ticker in Golang
Introduction to the time.Ticker package
This Golang package is a package that is used to repeat certain events which will continue to be repeated for a certain time. When this ticker has expired
it will send a trigger or signal to the channel.
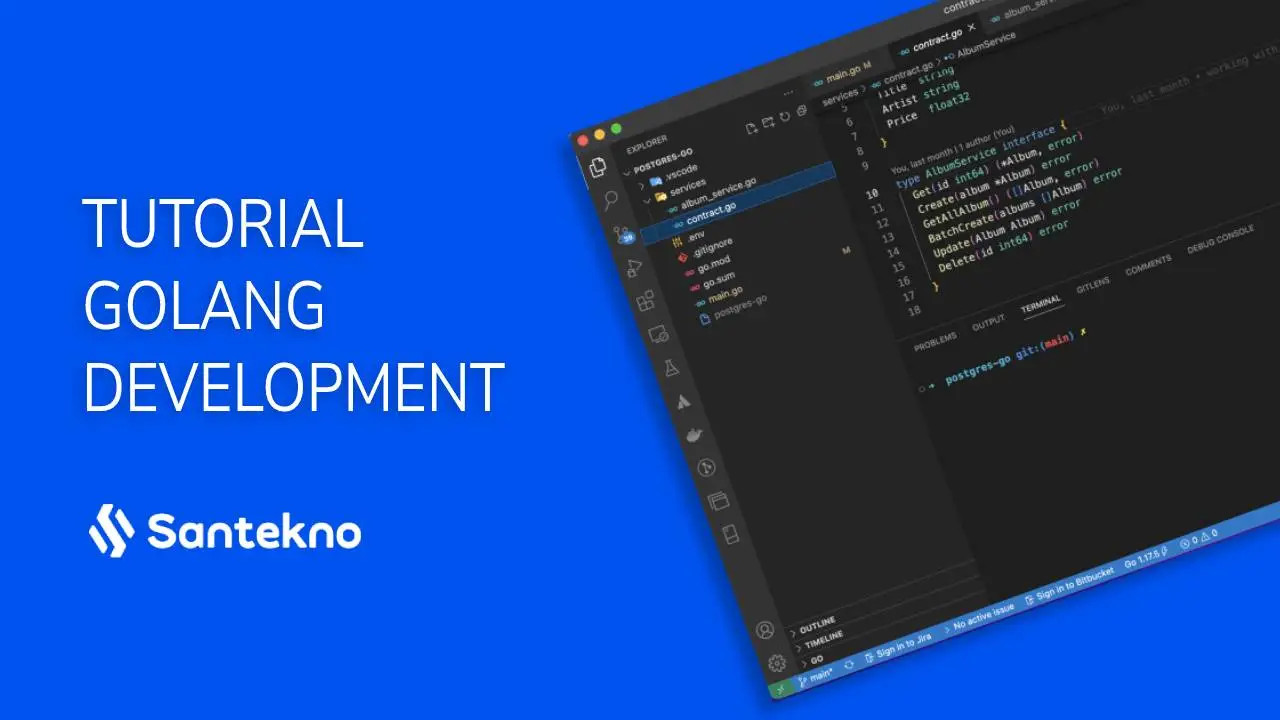
Get to know the Package Timer in Golang
Introduction to the time.Timer package
time.Timer
is a package that deals with times or events that will occur when the process is executed. For example, we will provide a ‘delay’ for a few seconds to go to the next process or there is another scheme.
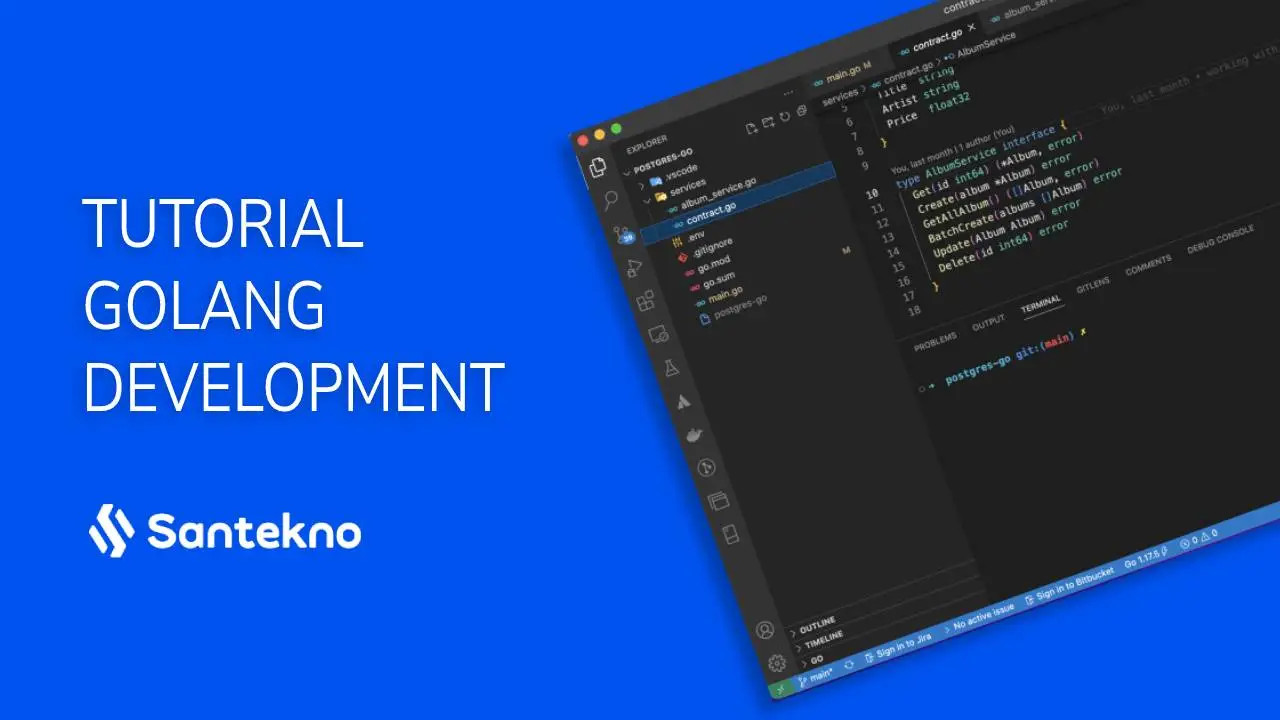
How to Overcome Handling Errors in Golang
Currently, Santekno will discuss error handling in the Golang language. We will learn from easy handling to some very complex implementations. Golang already provides easy error handling, we can also make slight modifications so that the error can be more easily understood by ourselves and the people who will collaborate with us in the future. The first thing we will do is customize Golang’s default Error
.
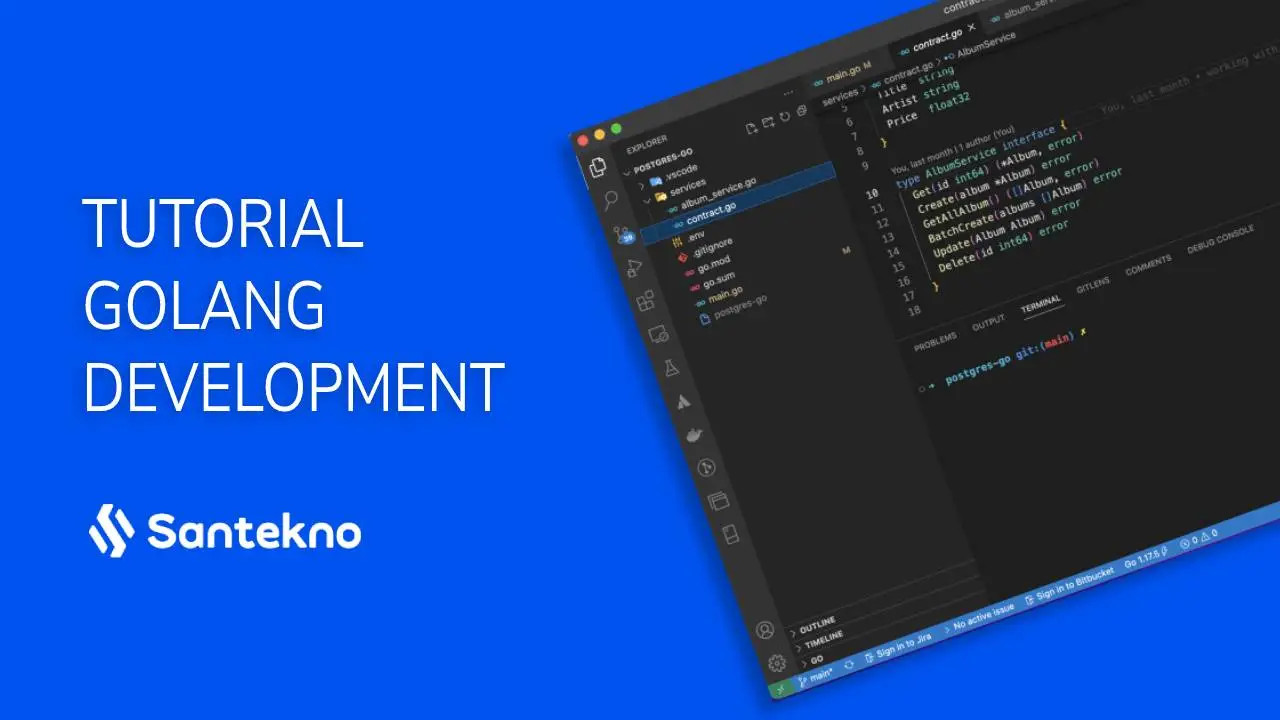
Techniques for Creating Mocking Unit Tests in Golang
When we create a function or code, sometimes we have difficulty carrying out unit tests at several points that we cannot cover with unit tests. So here are several technical ways to carry out unit tests using the mocking technique. But actually we can also use third-party which is already available in several libraries so we just need to use it straight away.
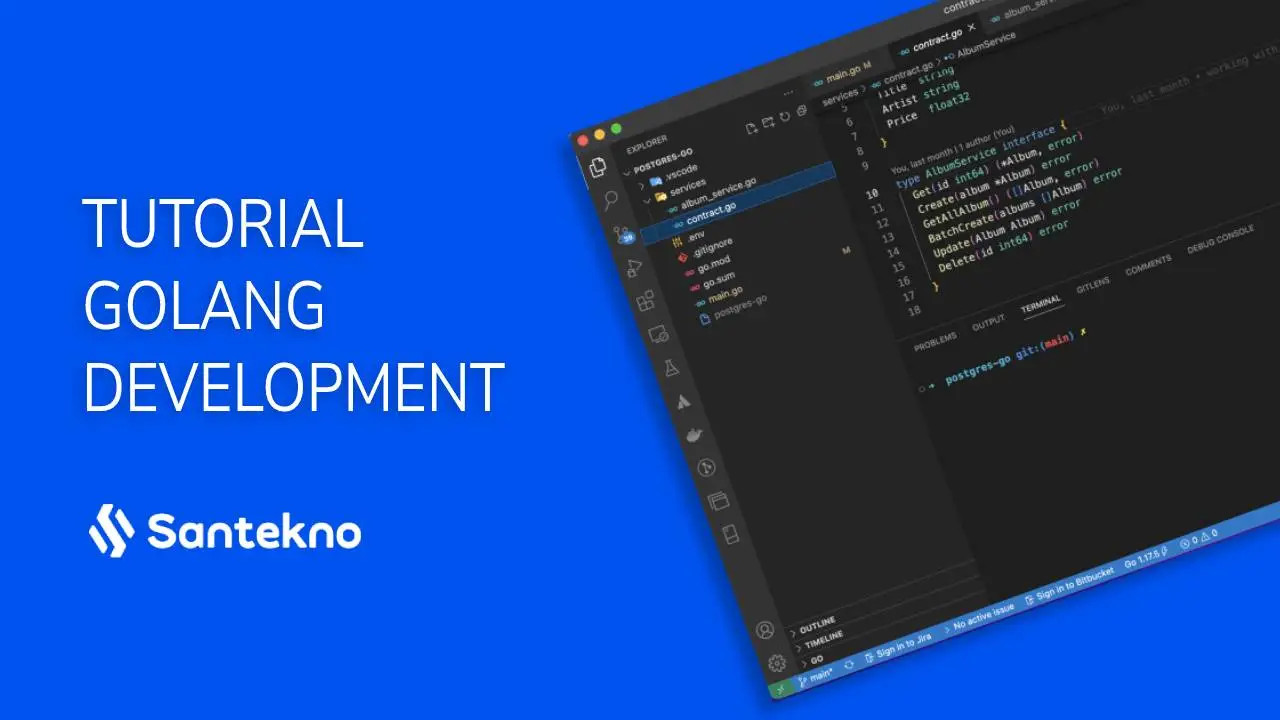
How to Create Integration Tests in Golang
Carrying out integration tests for APIs means that we must at least be able to run the application first so that integrated testing can be carried out.
We need to prepare several cases, test cases that cover the needs of the integration test. For example, the API Endpoint' that we have worked on has a
database,
cache` or other external resource that is related to the continuity of the API Endpoint.
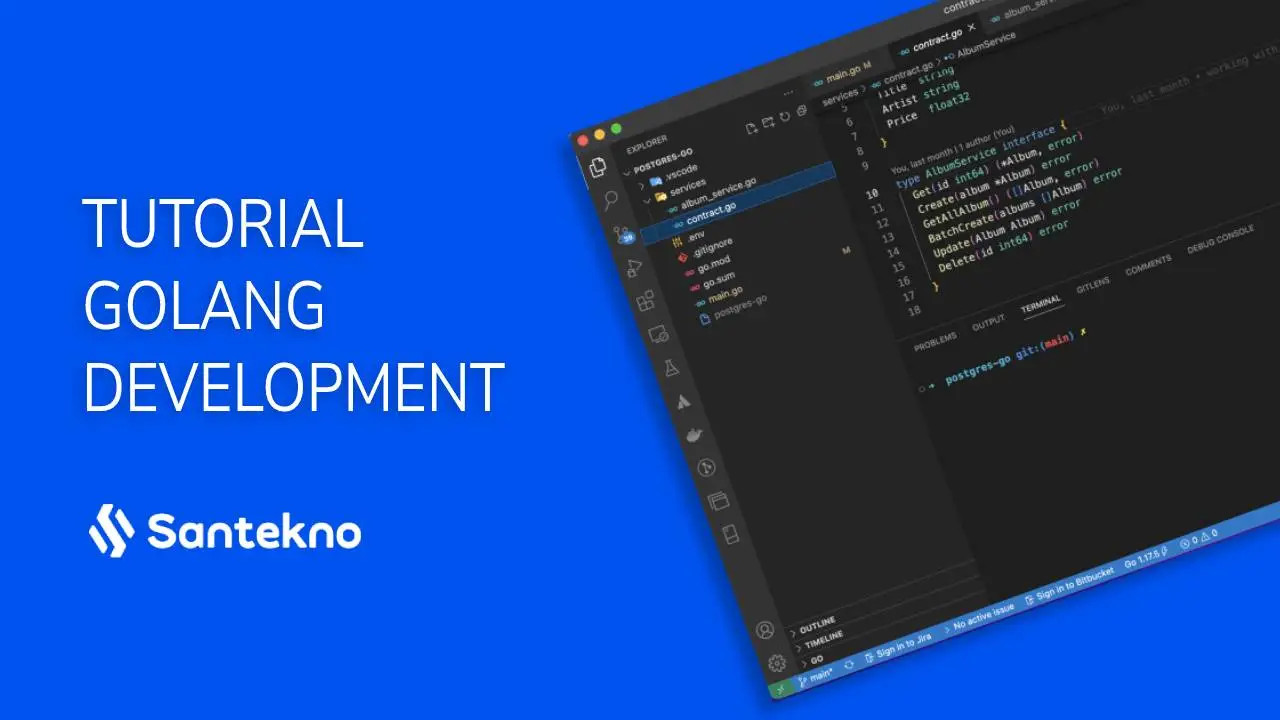
How to Create Unit Tests Using the moq Library in Golang
Carrying out unit tests using this mocking method is usually used if several functions have been carried out in interface
format so that we can assume that if we call the interface
function we believe that it should produce the correct program.
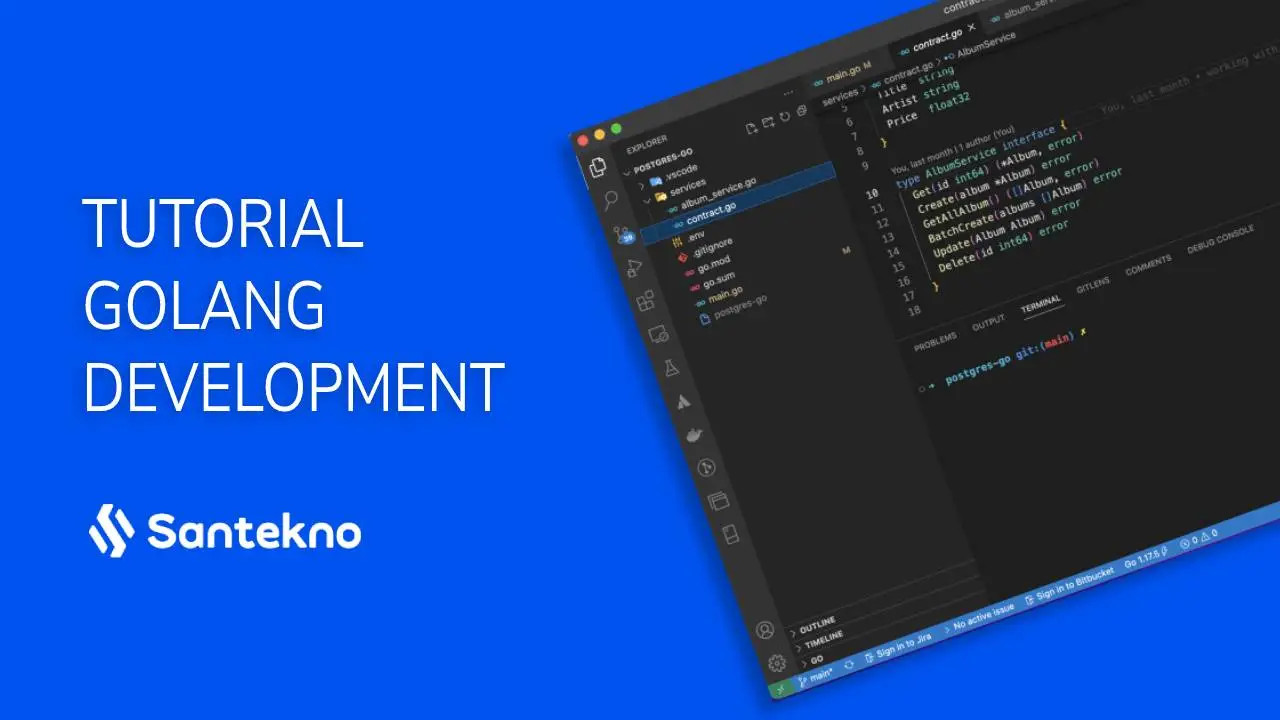
How to Create Benchmark Units in Golang
The testing
package in Golang Programming, apart from containing tools for testing, also contains tools for benchmarking. The way to create a benchmark itself is quite easy, namely by creating a function whose name begins with Benchmark
and whose parameters are of type *testing.B
.
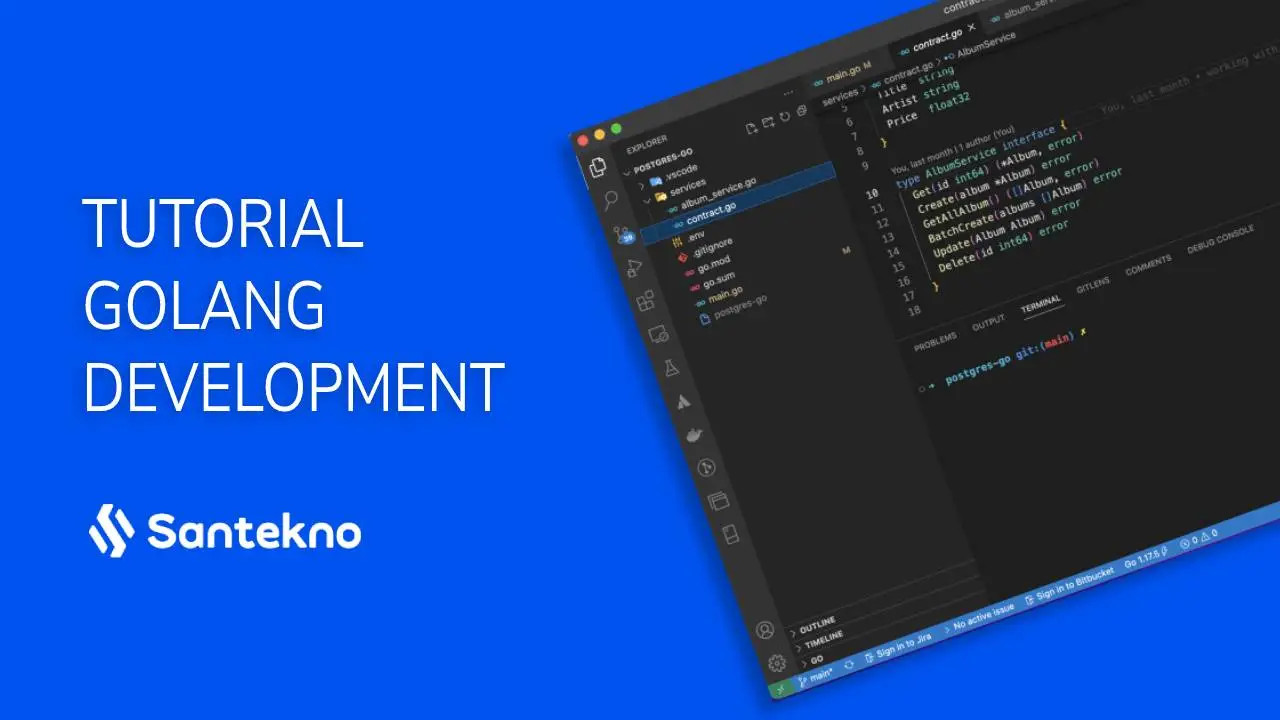
How to Create Unit Tests in Golang
Unit Testing Using the Go Library
programming is not easy, even the best programmers cannot write programs that work exactly as desired every time. Therefore, an important part of the software development process is testing. Writing tests
for our code is a good way to ensure quality and increase reliability.