#Golang-Web-Server
20 articles
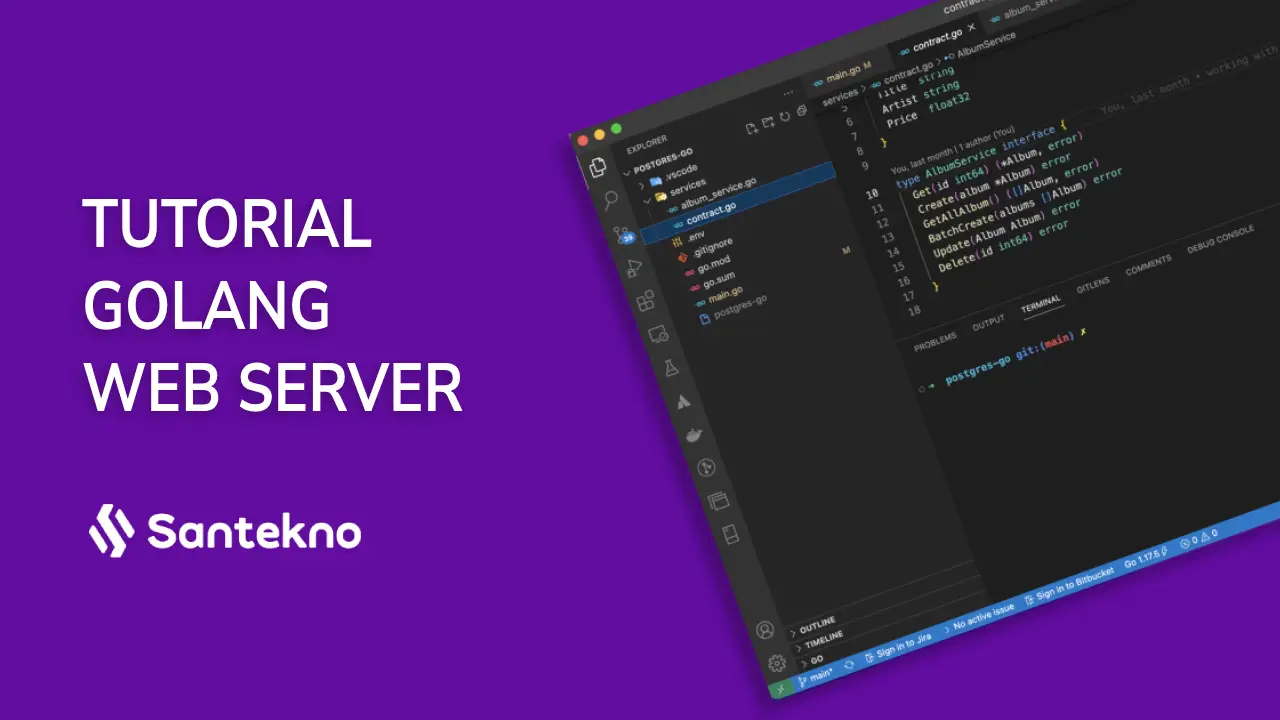
20 How to Understanding Routing Library in Golang
Routing Library
Golang actually provides ServeMux
as a handler that can handle several endpoints or the term is routing
. But most Golang programmers will usually use libraries to do this routing because ServeMux
does not have advanced features such as path variables, auto binding parameters and middleware. So there are many other alternatives that we can use for routing libraries besides ServeMux
.
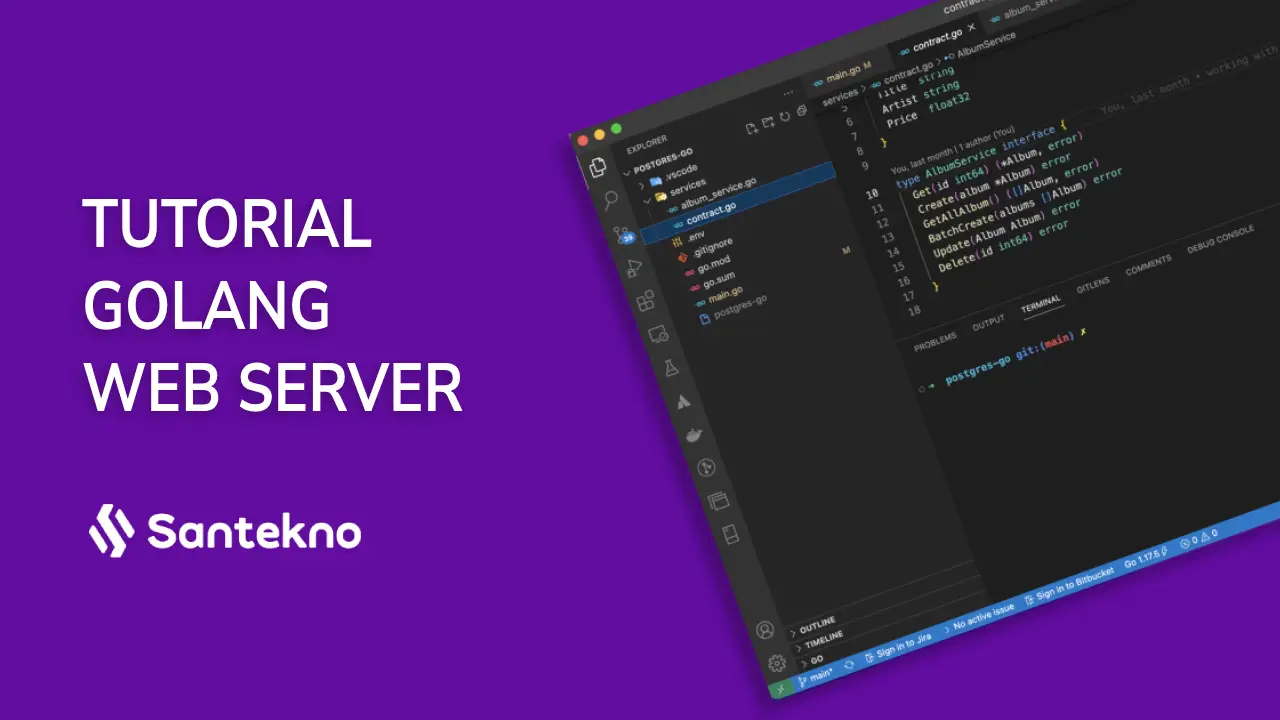
19 How to Understanding HTTP Middleware in Golang
Middleware
In web creation, we often hear the concept of middleware or filter or interceptor which is a feature that we can add code to before and after a handler is executed.
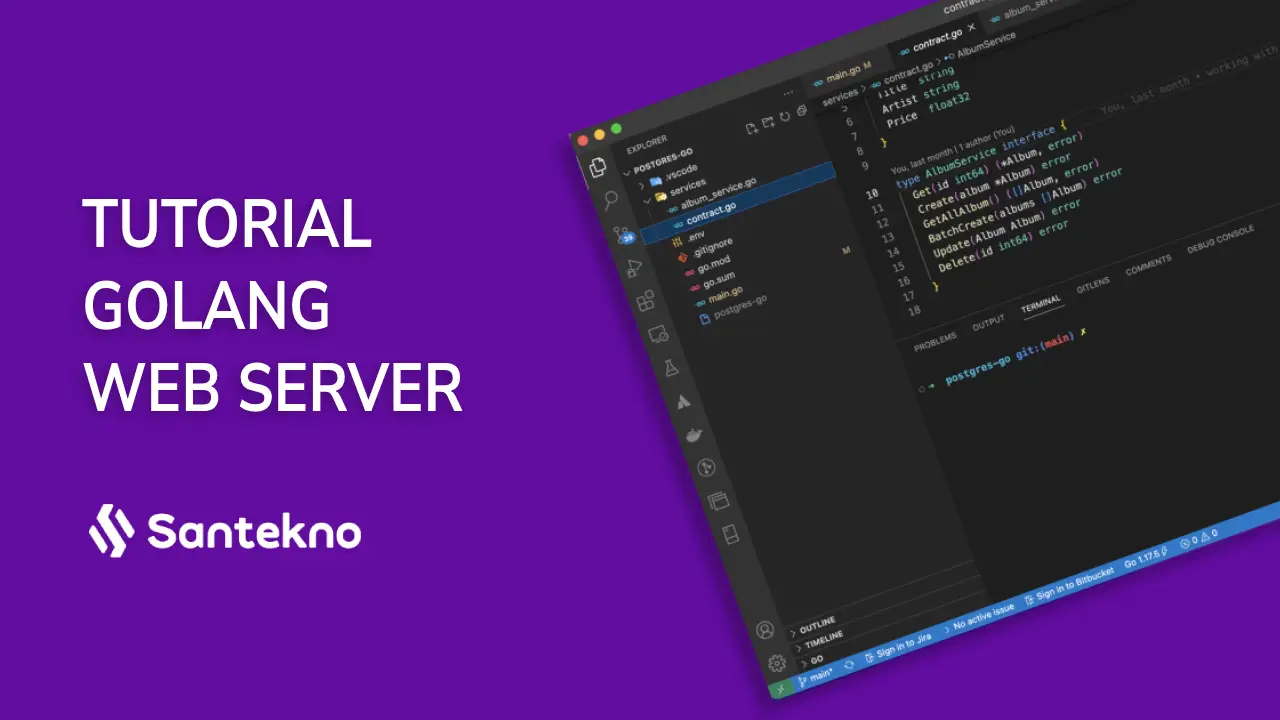
18 How to Download File in Golang
Download Files
Apart from uploading files, we also need or need a page that can download files or something on our website. The Golang library provides FileServer
and ServeFile
. If we want to force the file to be downloaded without having to be rendered by the browser then we can use the Content-Disposition
header. More details can be seen on this page https://developer.mozilla.org/en-US/docs/Web/Headers/Content-Disposition.
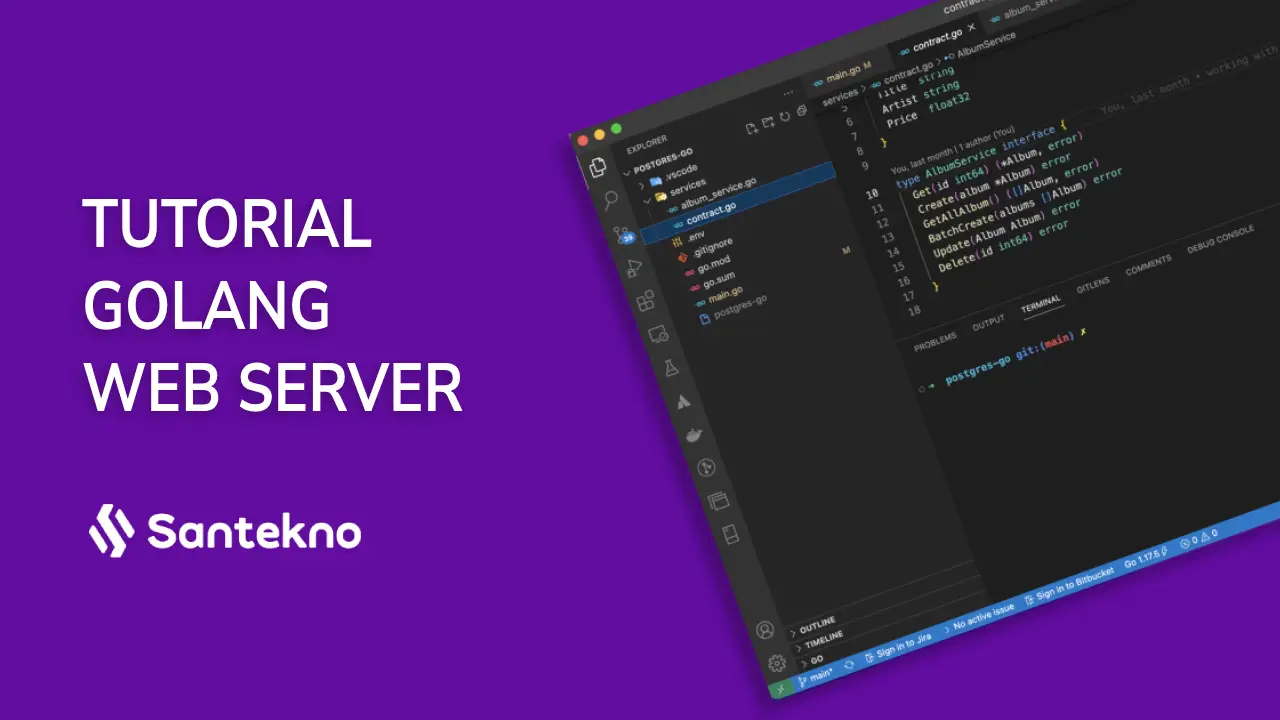
17 How to Upload File in Golang
Upload Files
Apart from receiving data input in the form of forms and query params, we usually also need data input in the form of files from our users. Golang actually already has this feature to handle file upload management. This makes it easier for us if we create a website that can accept file input.
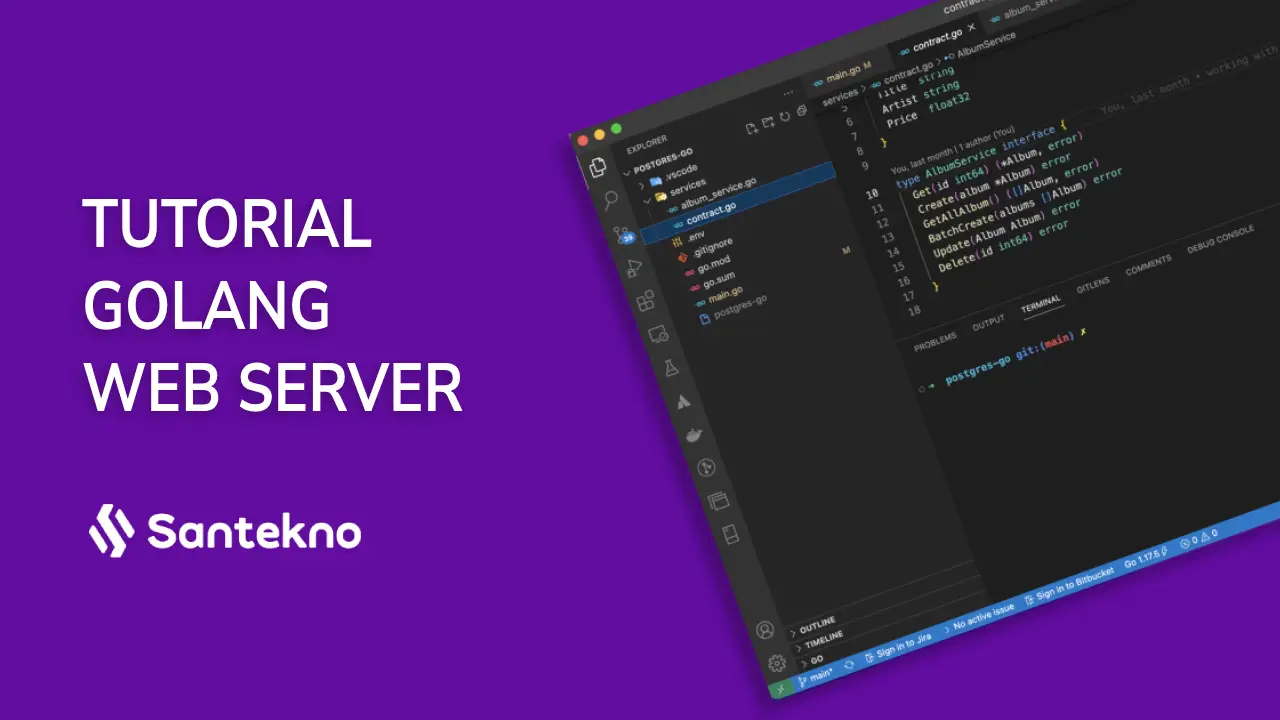
16 How to Understanding HTML Web Redirect in Golang
Redirects
When we create a website, if the user accesses various pages randomly, the page will not be found. So we need to ‘redirect’ a page if the user performs an action on our website page. For example, after logging in, we will redirect to the dashboard page. Redirect
itself is actually standard in HTTP and can be seen on the website page https://developer.mozilla.org/en-US/docs/Web/HTTP/Redirections. We only need to create a 3xx response code and add the destination location header. Luckily in Golang there is a function we can use to make this easier.
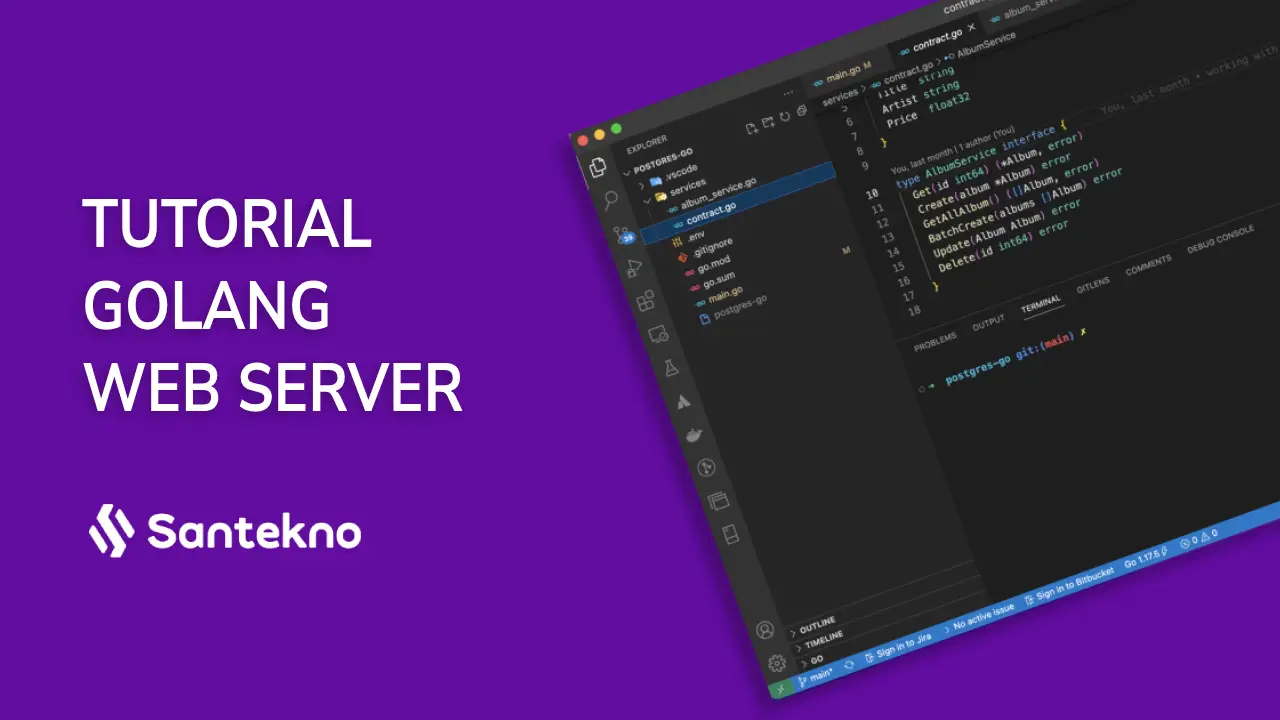
15 How to Understanding Web XSS (Cross Site Scripting) in Golang
Introduction to XSS (Cross Site Scripting)
XSS is one of the security issues that usually occurs when creating a website. XSS usually has security holes where people can deliberately enter parameters containing script or Javascript so that the website page can be rendered. The purpose of this XSS is to steal the browser cookies of users who are accessing the website and can cause user accounts to be taken over if we as website owners don’t handle it properly.
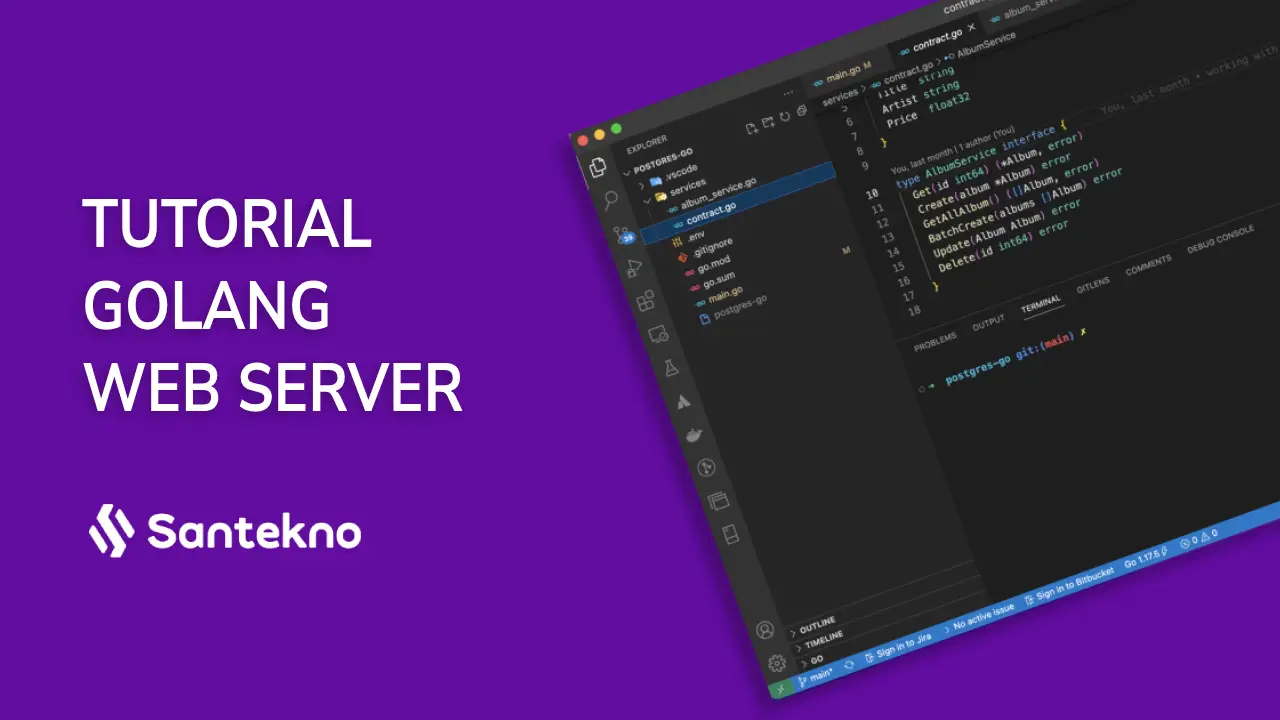
14 How to Understanding HTML Template Cache in Golang
Introduction to Cache Templates
In previous program codes that we have studied practically, they were not efficient. Why did it happen? because every time it is accessed it will call a function and describe the data sent into the template so the process requires high levels of execution. Every time the handler is called we will always re-parse the template. So ideally the template only performs parsing once at the beginning when the application is running, then only the template data is cached (stored in memory) so there is no need to access the data and do the parsing again so that the website we create can be faster.
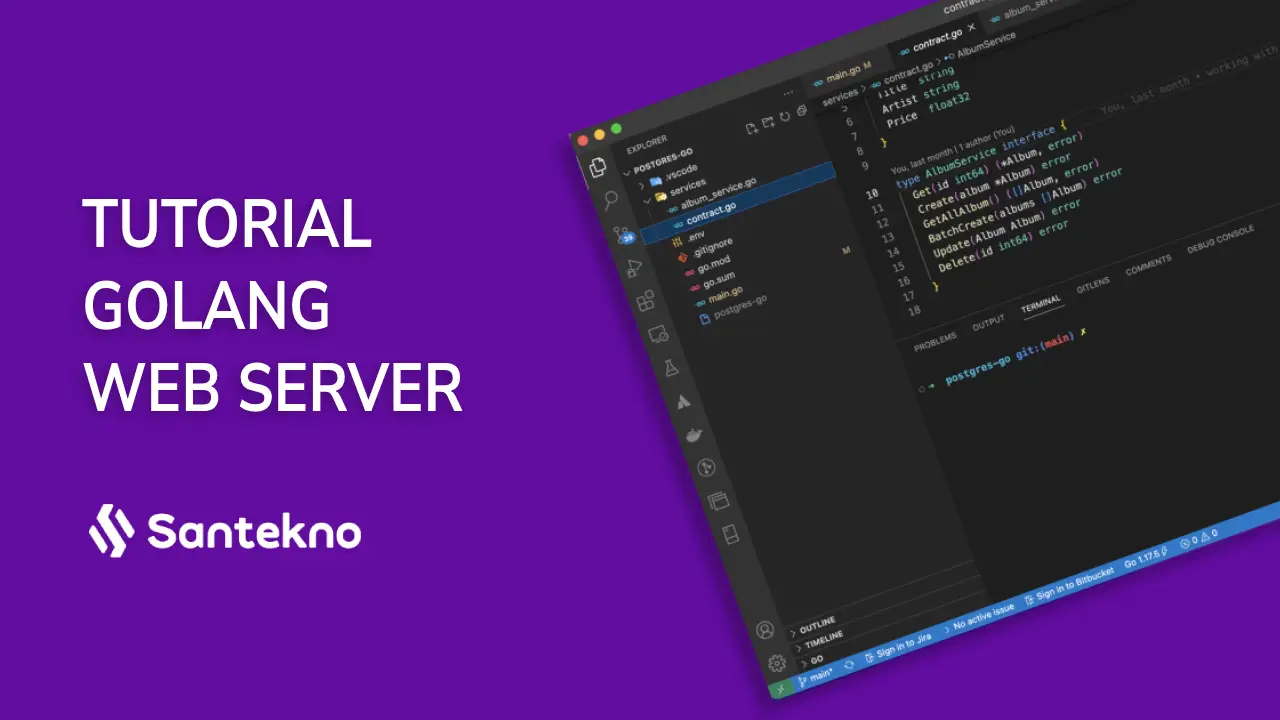
13 How to Understanding HTML Template Function in Golang
Introduction to Function Templates
Apart from accessing fields
in templates, we can also access functions or functions
in Golang. The way to access a function is the same as accessing a field
, but if the function has parameters then we can use additional parameters that are sent when calling the function in the template.
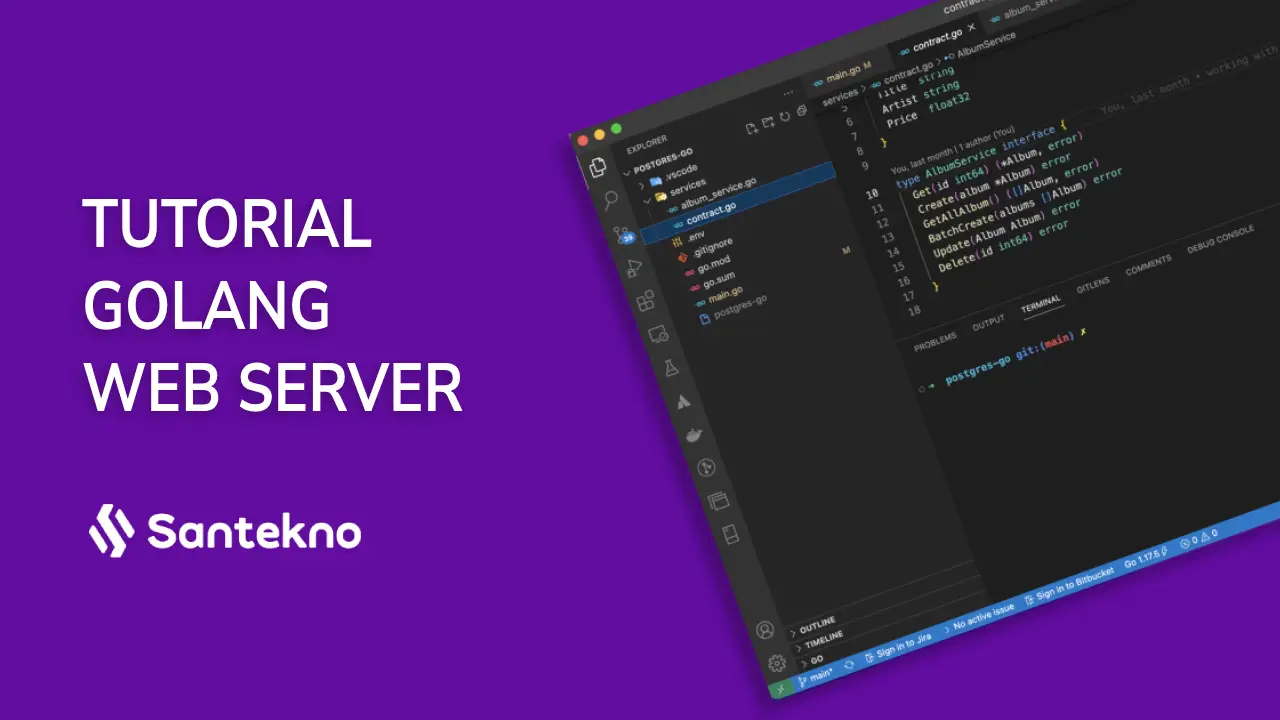
12 How to Understanding HTML Template Layout in Golang
Introduction to Layout Templates
When we create a website page, there are several parts that are always the same on each page, for example header
and footer
, so if there are parts that are the same it is recommended that they be saved in a separate template so that they can be used in other templates or sometimes we call them reusable
. Now, this Golang template supports importing from other templates so we can use it to make website creation easier.
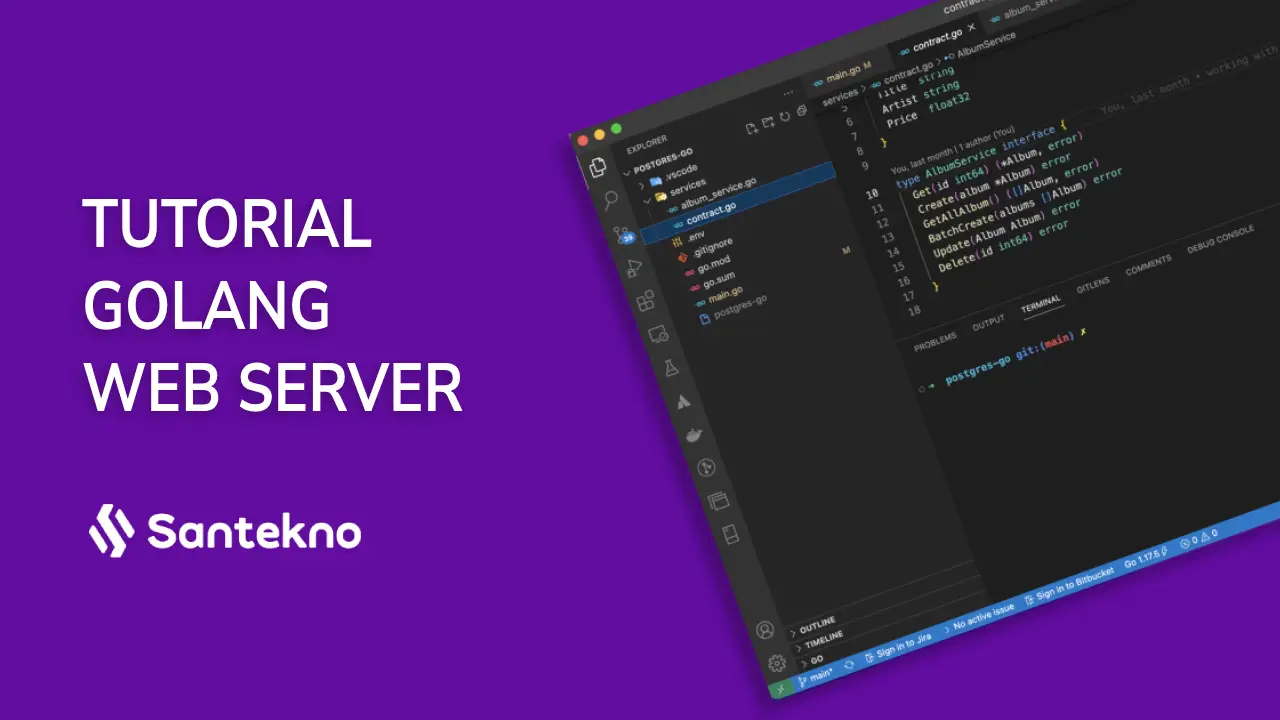
11 How to Understanding HTML Template Action in Golang
Introduction to Action Templates
Not only can we render text in templates, but we can also support action commands such as if
branching, for
loops and so on.
For example, suppose we use if
branching like this
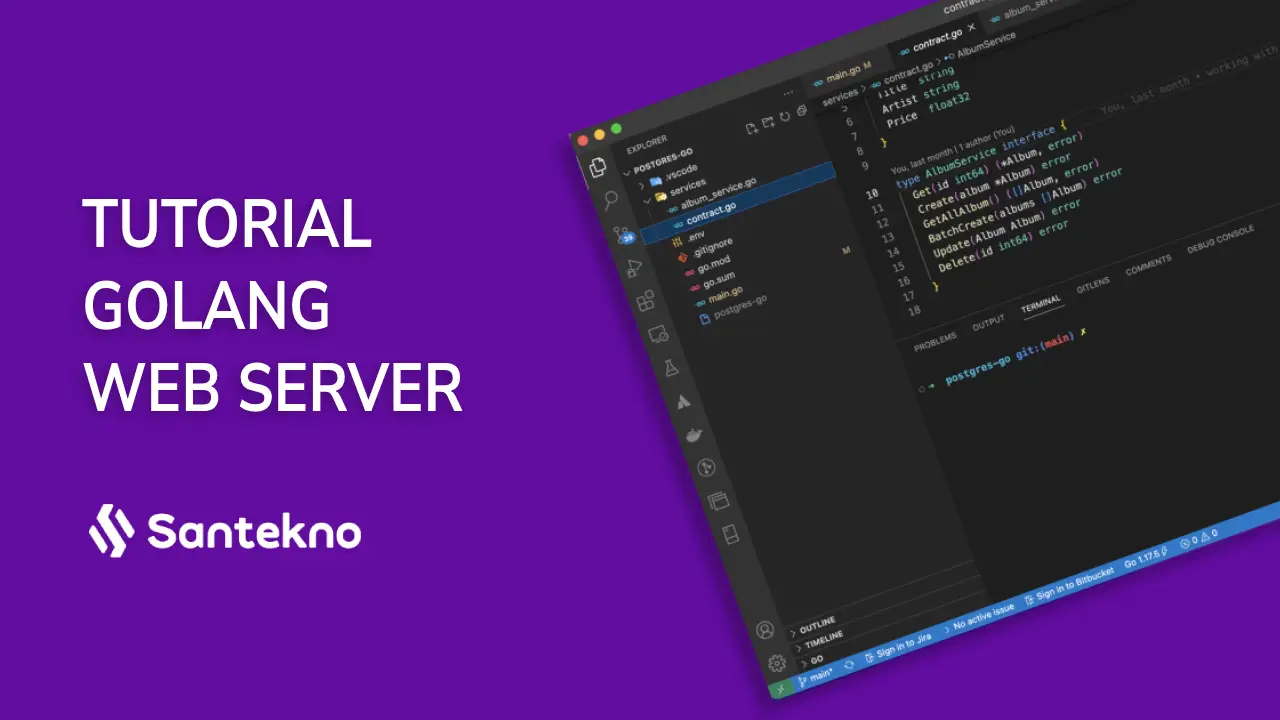
10 How to Understanding HTML Template Data in Golang
Introduction to Data Templates
If you have studied HTML Templates in the previous article, then we will continue with data templates where we can display this data dynamically by using struct or map data. However, we need to know the changes in the template text. We need to know the name of the field or key that we will use to fill in the dynamic data in the template. We can mention the name of the field
or key
, for example {{.FieldName}}
.
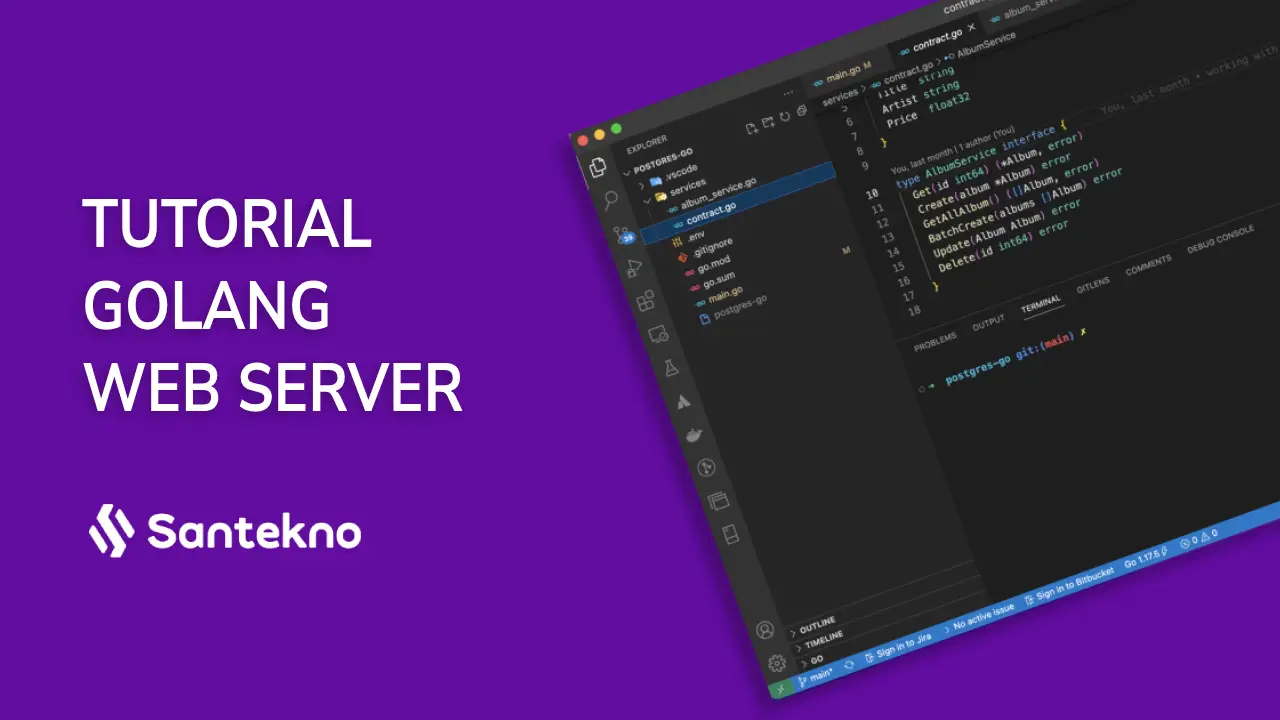
09 How to Understanding HTML Template in Golang
Dynamic Web using Templates
In the previous post we discussed dynamic websites but using response strings contained in static files. So on this occasion we will try to get to know templating in Golang where the page, say HTML, will be dynamic and can change with the data accessed by the user.