#Web
49 articles
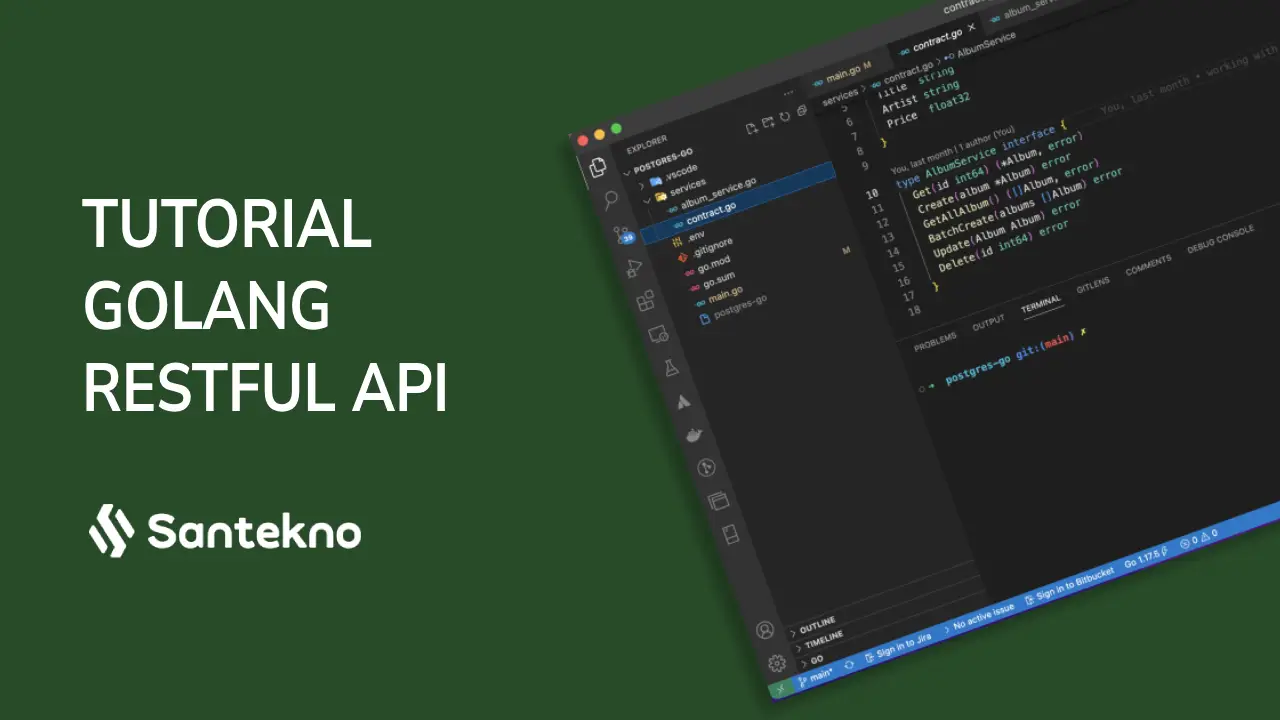
12 Creating Dependecy Injection Library Google Wire
This time we will try to make Dependency Injection using the Wire Library from github.com/google/wire. The usefulness of this library is that we can make so many dependencies that we can generate directly concisely and easily.
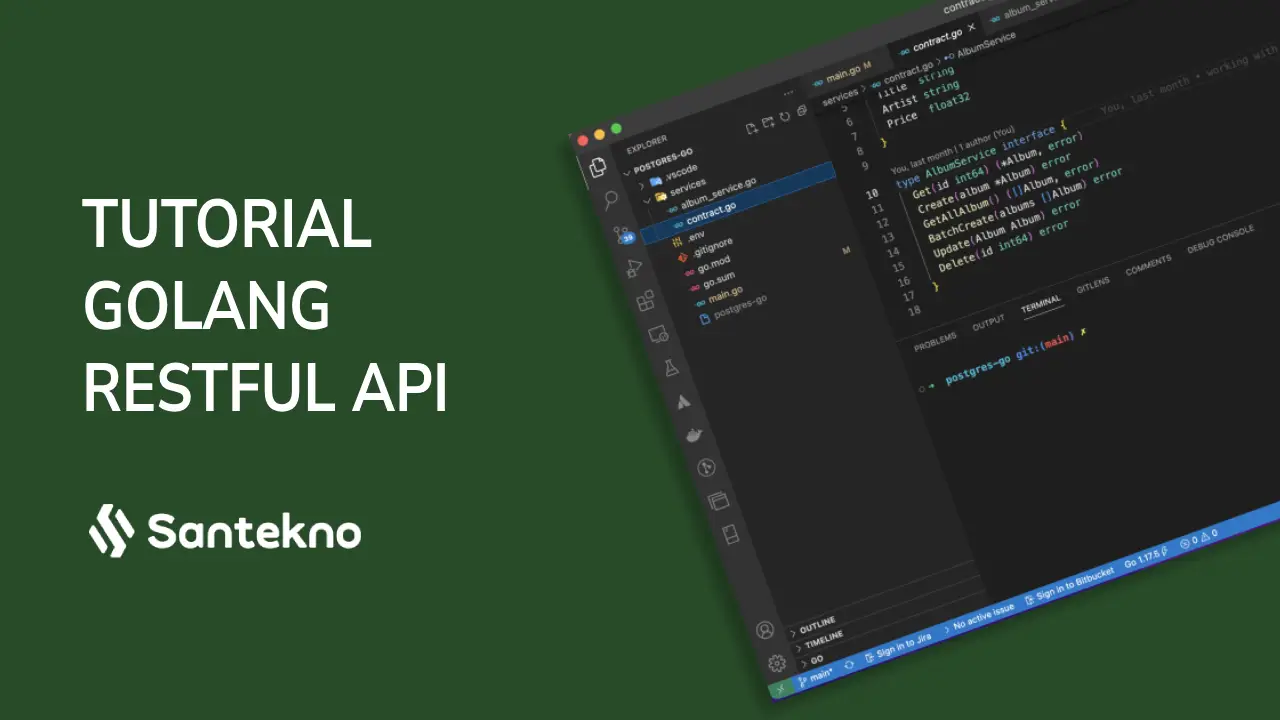
11 Add Unit Tests Using Mockery
At this stage we will need the following dependencies
brew install mockery
go get github.com/stretchr/testify
go mod tidy
go mod vendor
go get github.com/DATA-DOG/go-sqlmock
In this project we will try to create unit tests using a mocking library with the name mockery
. This library is widely used by golang developers because of its ease of use and has the advantage of features that can cover all the necessary unit tests. If you have used unit tests in golang, you may already know this library but if you want to get more references, Santekno has also provided previous posts including:
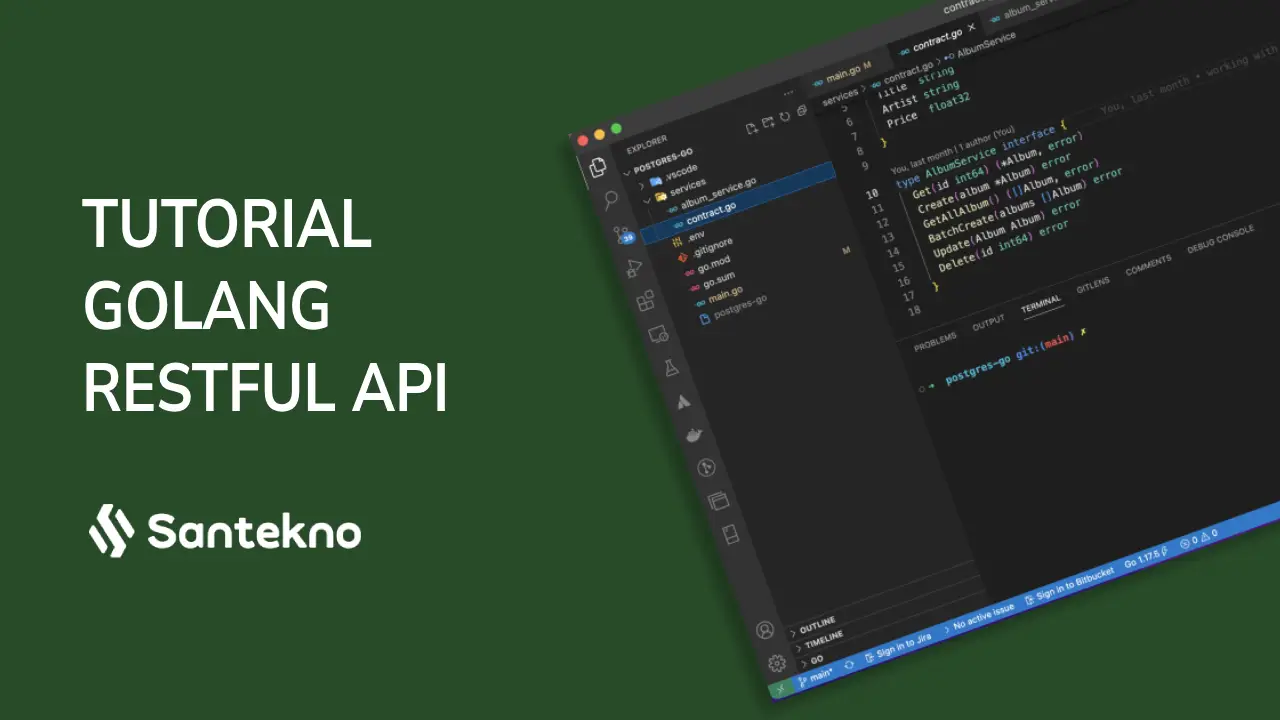
10 Adding Simple Authentication
At this stage we will try to add simple Authentication by using middleware in Golang. You need to know that middleware is the process of intercepting an API which before the service when accessed goes to the *handler * layer, it will pass through this * middleware * layer (which we will create) to capture and process something for certain needs. For example, in this case we will intercept the service process to see if the API has a header with the key condition X-API-Key
.
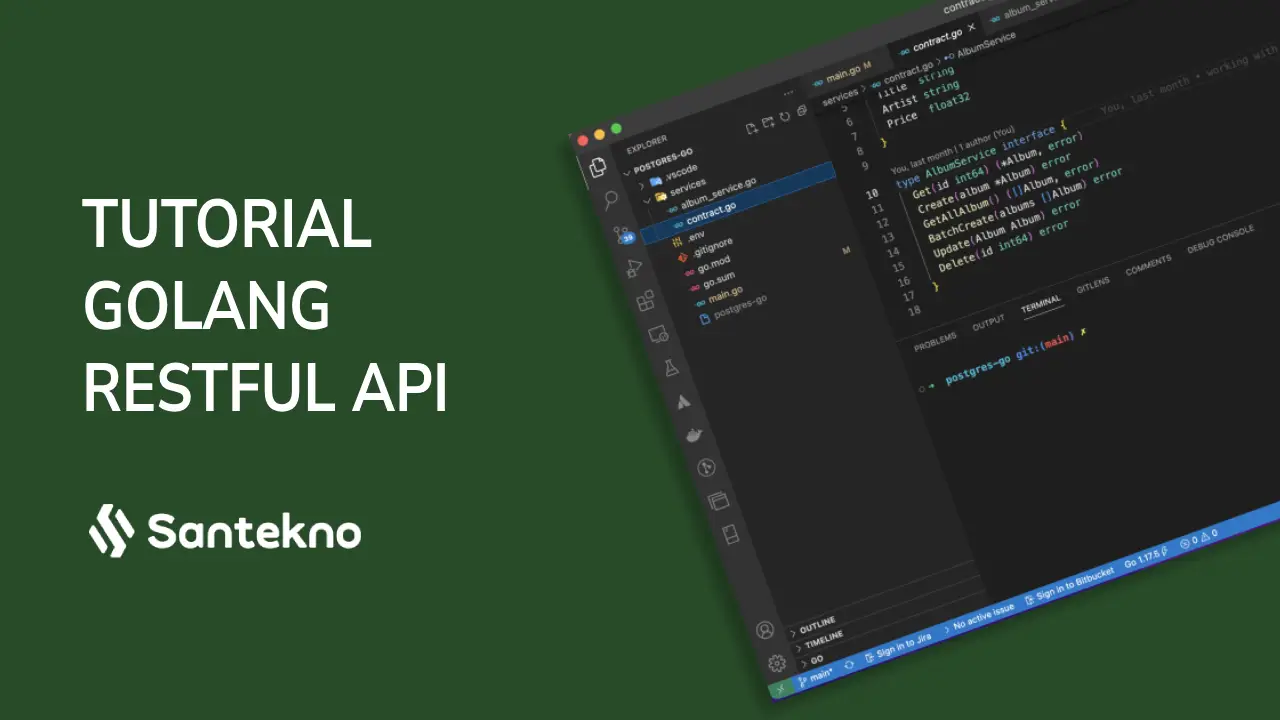
09 Configuring HTTP Router, HTTP Server and Database Connection
At this stage we will create the main
function of the project that we have created. In the main
function we will add several function initializations which are used to initialize all the resources
needed by the project such as database connections, handler initialization, usecases and repositories that we have previously created.
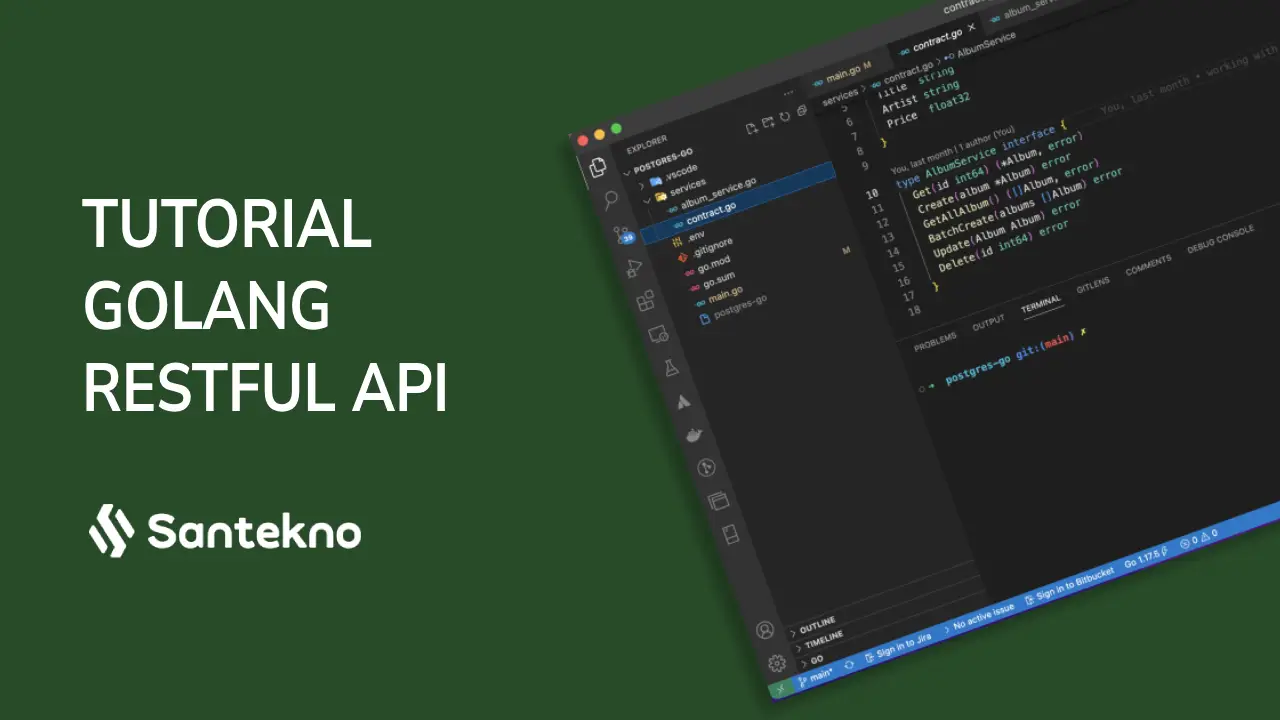
08 Adding a Request Validator Using Golang Playground
At this stage we will add validation for each request sent to the API Application on our Services for example on add, change and delete requests. The validation we use is package github.com/go-playground/validator.
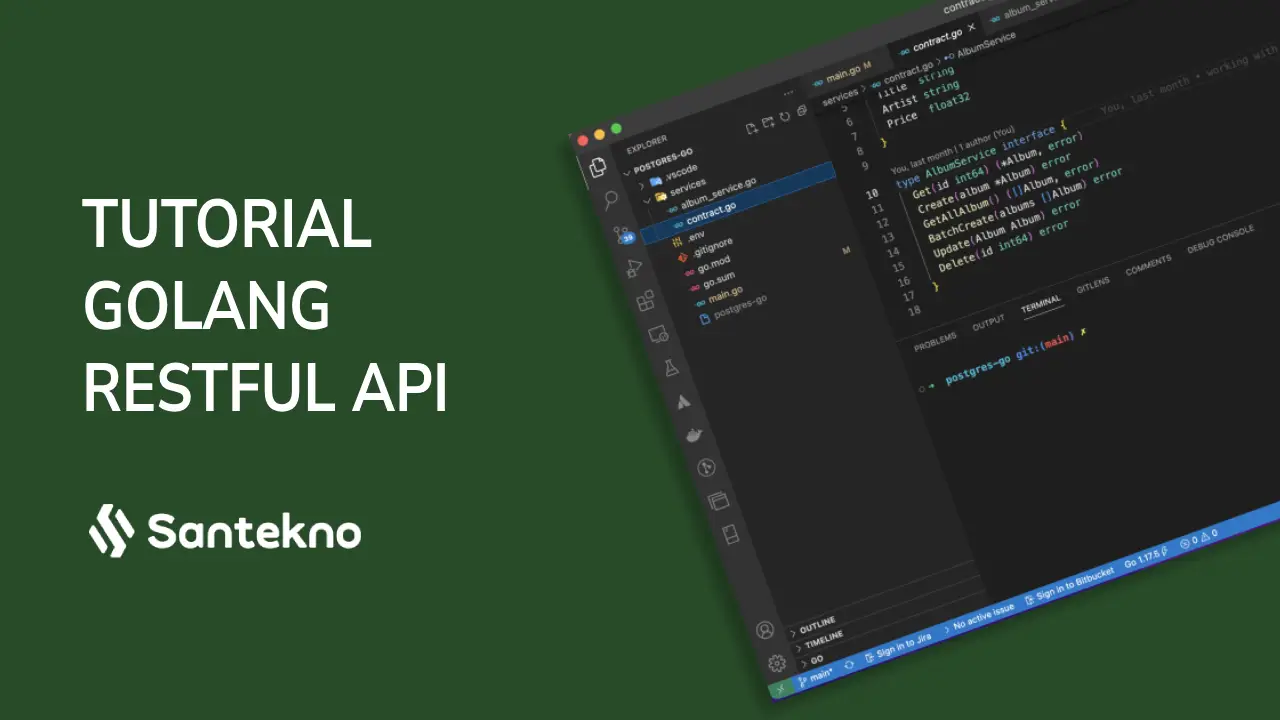
07 Creating a Handler as a Data Delivery Layer
At this stage we will continue the project that we have created by creating a Delivery Layer. This layer as explained earlier is a layer that connects user requests with the logic layer.
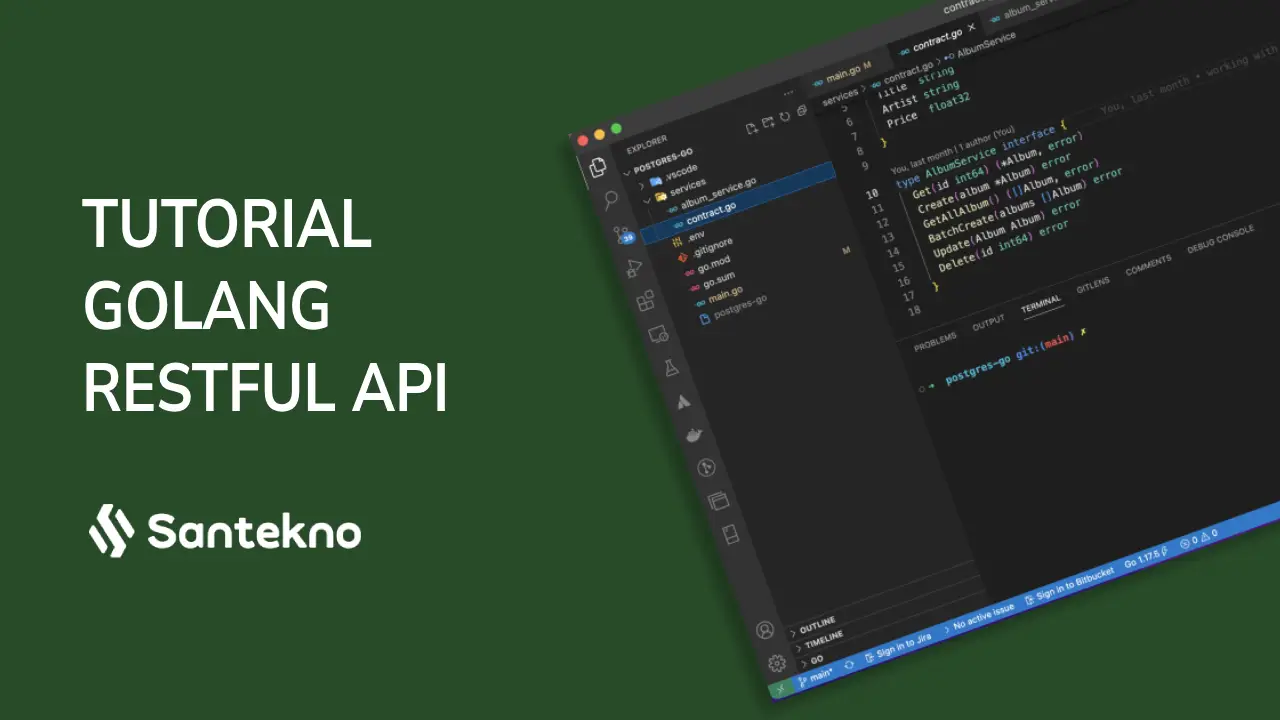
06 Creating Usecases as Data Logic
This time we will continue the project of making this RESTFul API by creating a Usecase function. Previously it was discussed that this Usecase Layer will contain logic data or data processing which is used some logic needed in a process.
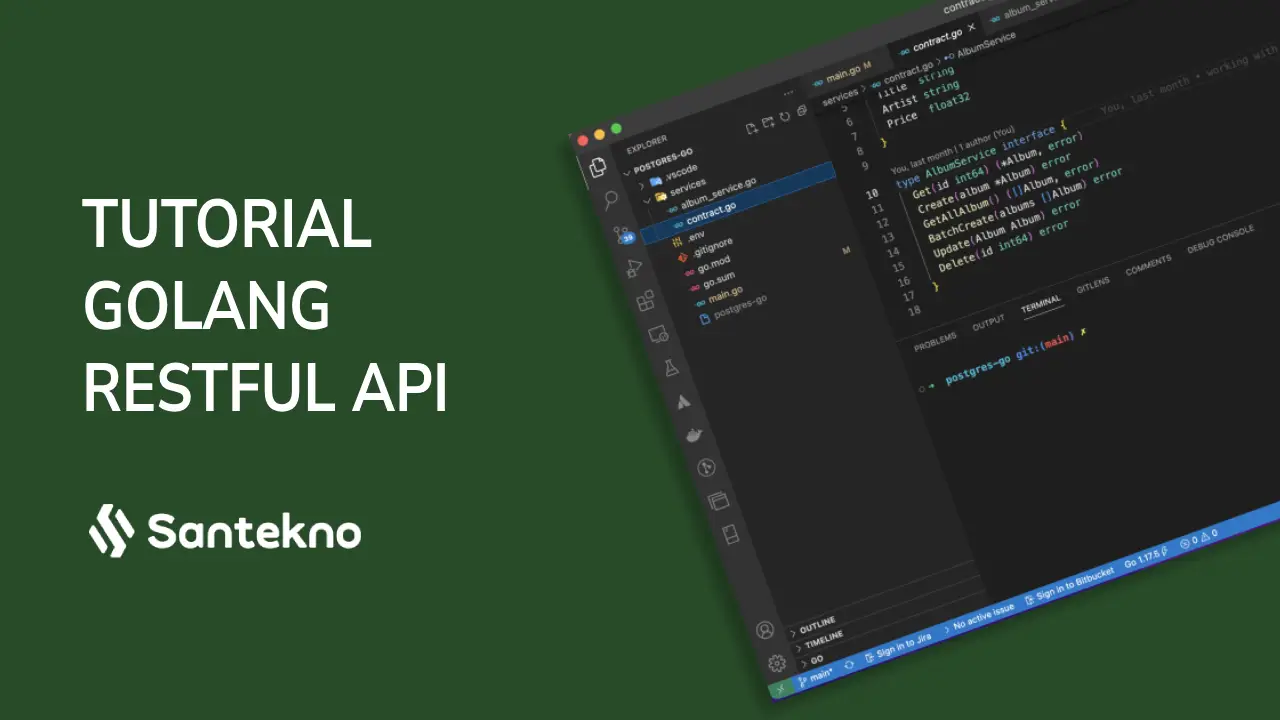
05 Creating a Repository as Access Data
This time we will create an Article Repository that needs to communicate to the MySQL database. We have a table in MySQL previously explained, then we will create functions that will be used for API specification needs. Here are the functions that we will like this
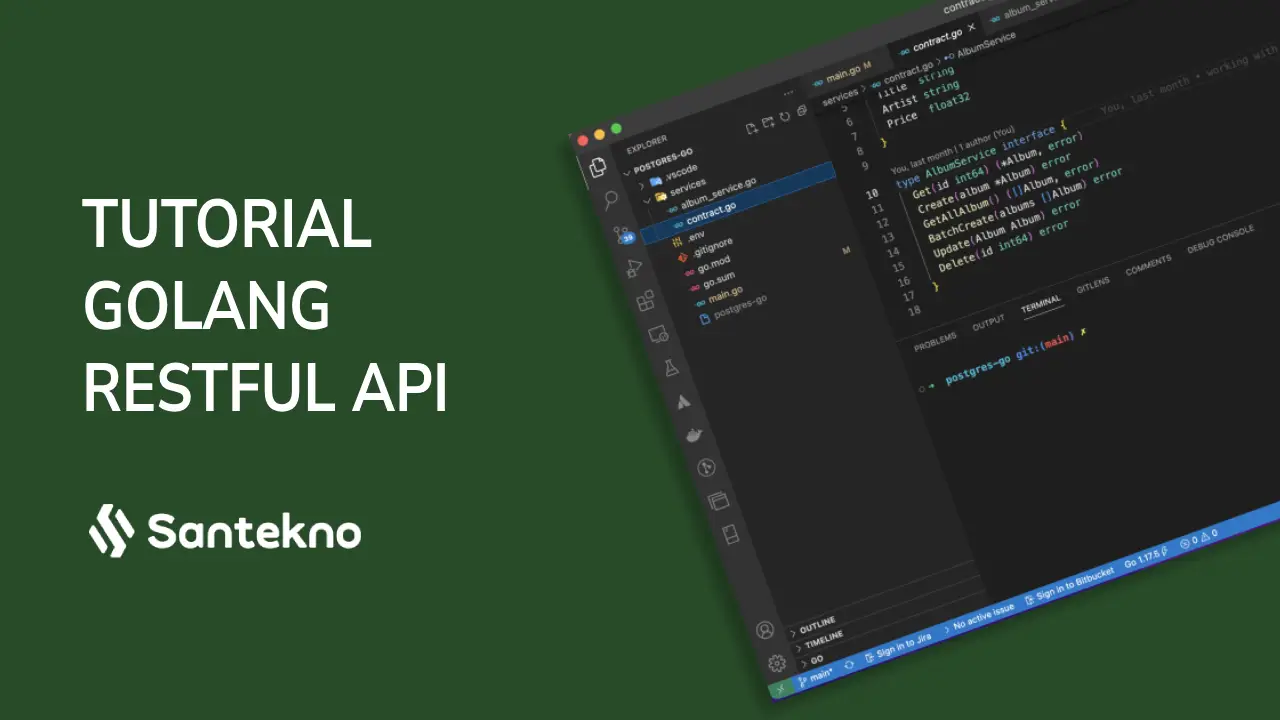
04 Implementing Clean Architecture on Project
At this stage we will try to implement Clean Architecture, where this concept according to Uncle Bob has 4 layers, namely
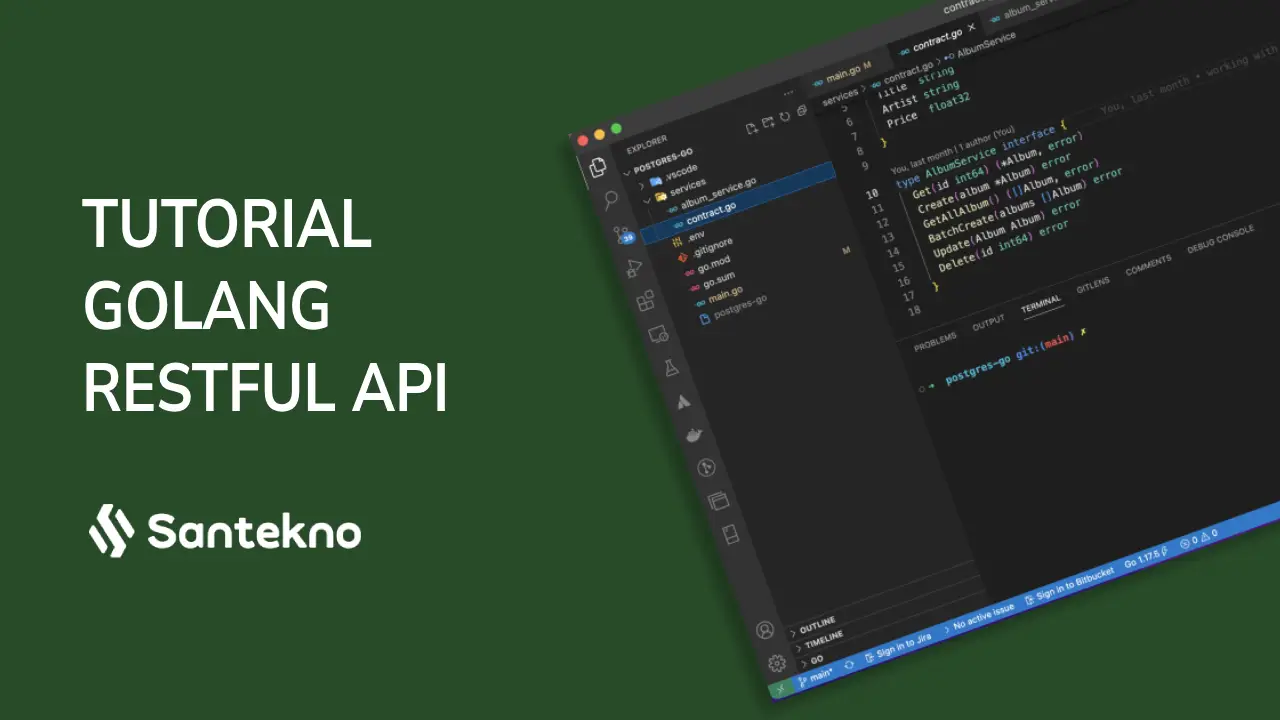
03 Creating a Database on MySQL Golang
At this stage we will try to create a database on MySQL in preparation for creating the table that we will also use later to create an API with the data in the database. Previously, if you didn’t have MySQL on a computer or laptop, prepare to install MySQL and the Database Editor first, you can use DBeaver, MySQL Workbench or what you usually use for database management.
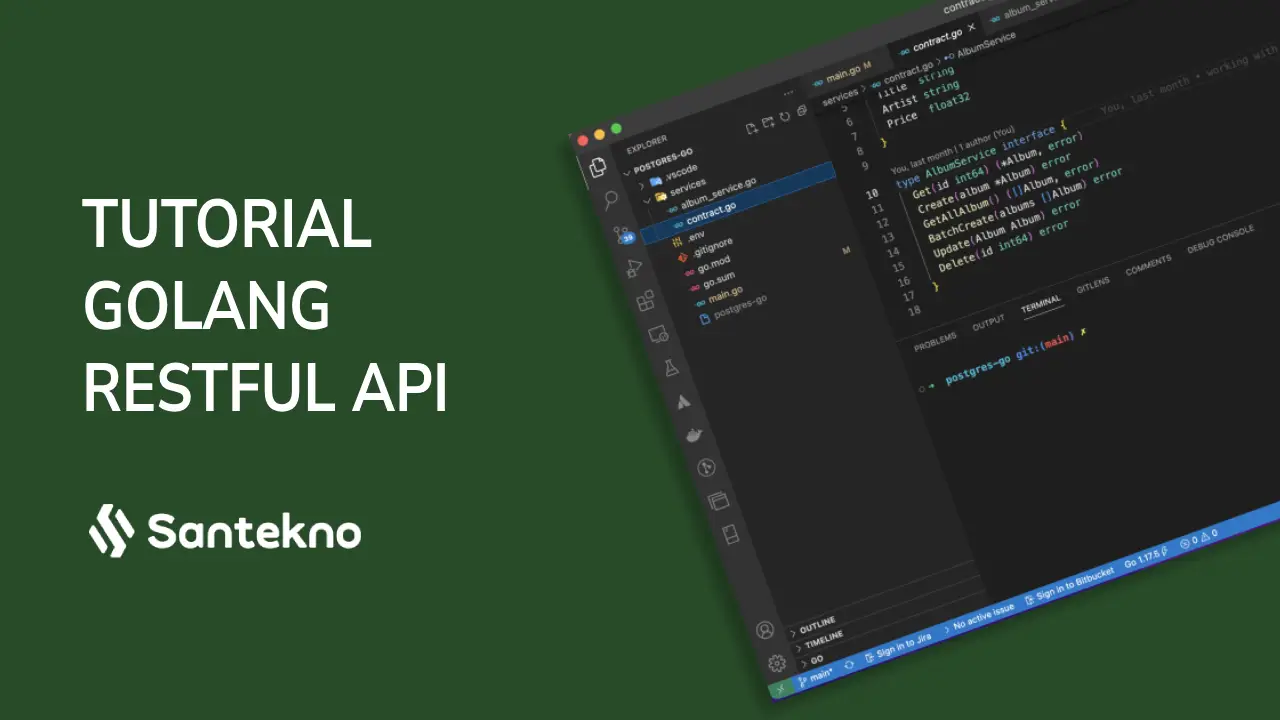
02 Membuat API Specification menggunakan OpenAPI
At this stage we will try to create all the API Specifications related to the API that we will create so we need complete documentation using OpenAPI. If you haven’t installed OpenAPI in the repository, try reading the previous article first.
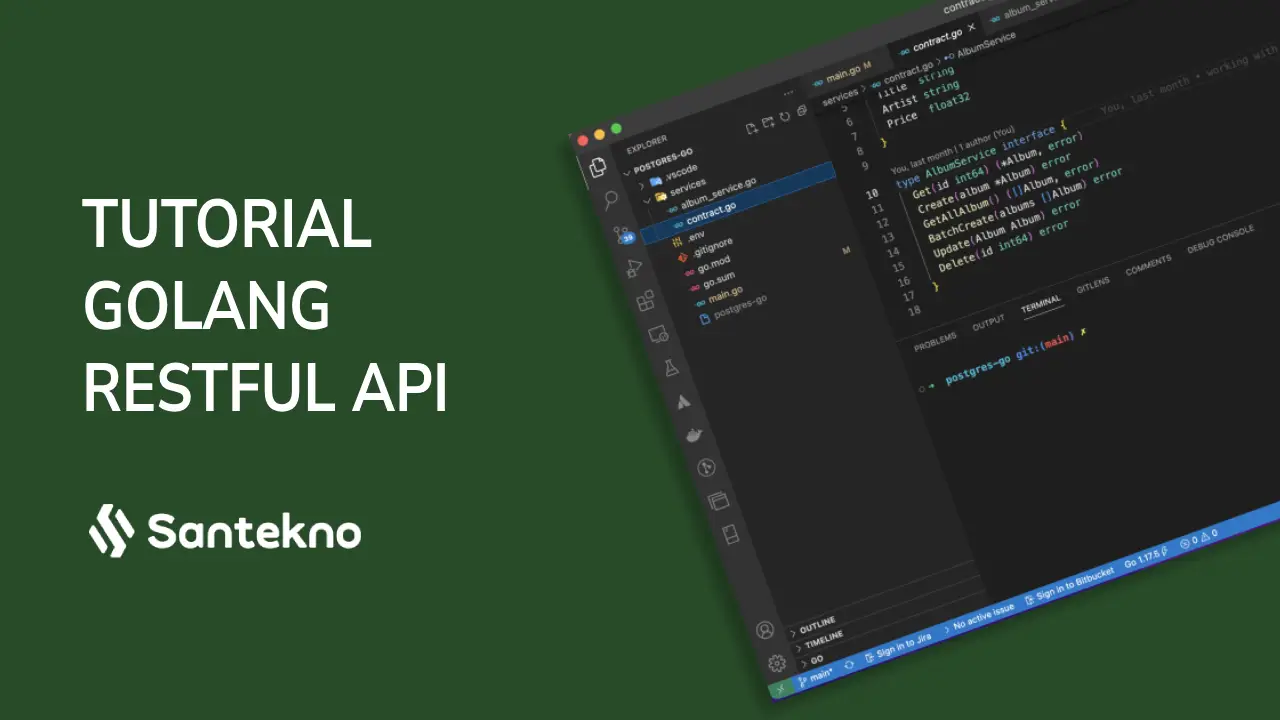
01 RESTful Introduction to Golang
Introduction to RESTFul API
Quoted from the amazon website, RESTful API is an interface used by two computer systems to securely exchange information over the internet. Most business applications must communicate with other internal and third-party applications to perform various tasks. For example, to generate monthly payslips, your internal accounts system must share data with your customers’ banking systems to automate billing and communicate with internal time and attendance applications. RESTful APIs support this exchange of information because they follow software communication standards that are secure, reliable and efficient.